Here you will find a brief JUnit Tutorial.
Getting Started With JUnit: A Beginner’s Guide. An introductory article that explains what JUnit is, its importance in software testing, and how to set it up in a Java project.
Writing Your First JUnit Test. A step-by-step guide on writing a simple JUnit test case. Cover the basic annotations like @Test
, @Before
, and @After
.
Parameterized Tests in JUnit. It covers how to write parameterized tests in JUnit, where you can run the same test with different input values.
Best Practices for Writing JUnit Tests. Here you will get tips and best practices for writing effective and maintainable JUnit tests, including naming conventions, test structure, and assertions.
JUnit Test Suites. Here we describe how to create and use test suites in JUnit to organize and run multiple test classes together.
Mocking with JUnit. In this article, we will explore how to use mocking frameworks like Mockito in combination with JUnit to isolate and test specific components of your code.
JUnit 5 Features and Upgrades. Here you will get an overview of the new features introduced in JUnit 5, including parameterized tests, nested tests, and dynamic tests.
Integration Testing with JUnit. Here, we will explain how to perform integration testing using JUnit, covering topics like setting up a test database, testing web services, and more.
Continuous Integration with JUnit. How to integrate JUnit tests into a CI/CD pipeline, such as Jenkins or Travis CI, to automate testing as part of the development workflow is discussed here.
Testing Spring Boot Applications with JUnit. A guide on using JUnit to test Spring Boot applications, including integration testing of Spring components.
Test Driven Development (TDD) with JUnit. We will explore how to practice Test Driven Development using JUnit, where tests are written before the actual code.
JUnit and Code Coverage. How to measure code coverage using tools like JaCoCo in conjunction with JUnit tests is discussed here.
Advanced JUnit Techniques. It covers advanced topics like rule-based testing, custom test runners, and custom annotations in JUnit.
JUnit vs. TestNG. Compare JUnit with another popular Java testing framework, TestNG, highlighting their differences and when to use each.
JUnit Best Practices for Android Development. If you’re into Android development, write about JUnit best practices specific to Android app testing.
Troubleshooting JUnit Tests. Common issues and solutions that developers may encounter while writing and running JUnit tests are shared here.
Testing Microservices with JUnit. Strategies and best practices for testing microservices architecture using JUnit are discussed here.
Jenkins Pipeline for JUnit Tests. A detailed guide on setting up Jenkins pipelines for running JUnit tests automatically.
JUnit Test Reporting and Visualization. How to generate and interpret test reports in JUnit, including HTML reports and visualization tools is explained here.
JUnit and Test-Driven Development (TDD) Case Study. A walk through of a real-world case study of applying JUnit and TDD to solve a specific programming problem.
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
100+ MCQs On Java Architecture
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
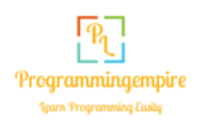