In this article, we will describe JUnit 5 Features and Upgrades.
JUnit 5 Features and Upgrades- A Brief Overview
JUnit, the popular Java testing framework, has undergone significant improvements with the release of JUnit 5. This version introduced several new features and upgrades to make unit testing in Java more powerful, flexible, and enjoyable. In this post, we’ll explore the key features and improvements that JUnit 5 brings to the table.
1. Modular Architecture
JUnit 5 embraces a modular architecture, breaking the framework into different modules to offer flexibility in selecting and using only the components you need. The core modules include junit-jupiter
(for JUnit 5 tests), junit-platform
(for running tests), and junit-vintage
(for running JUnit 3 and 4 tests). This modular approach allows developers to customize their testing setup better.
2. JUnit Jupiter
Additionally, JUnit 5 introduces a new programming model called JUnit Jupiter. In fact, it’s designed to address the limitations of JUnit 4 and provides many new features:
a. Annotations
JUnit Jupiter introduces new annotations like @Test
, @BeforeEach
, @AfterEach
, @BeforeAll
, and @AfterAll
. Basically, these annotations offer more expressive and fine-grained control over your test methods and setup/teardown logic.
b. Nested Tests
Further, JUnit 5 allows you to define nested test classes, which improves test organization and makes it easier to group related tests together. This is especially useful for testing complex components with multiple levels of nesting.
c. Dynamic Tests
Dynamic tests enable you to generate and run tests at runtime, which is incredibly handy for parameterized testing and testing scenarios where the number of tests isn’t known beforehand.
d. Extensions
Also, JUnit Jupiter introduces the concept of extensions, which replace the need for custom runners and rule chains in JUnit 4. Basically, extensions provide reusable, composable, and context-aware functionality for your tests.
3. Improved Assertions
Indeed, JUnit 5 enhances its assertion capabilities with the introduction of the assertAll
method, which allows you to group multiple assertions together. As a result, if one assertion fails, all the assertions within the group are executed, providing a more comprehensive view of test failures. The following code shows this.
@Test
void testMultipleAssertions() {
assertAll(
() -> assertEquals(2, add(1, 1)),
() -> assertEquals(0, subtract(2, 2)),
() -> assertEquals(4, multiply(2, 2)),
() -> assertEquals(3, divide(9, 3))
);
}
4. Improved Test Reporting
Likewise, JUnit 5 offers better test reporting, including a more detailed summary of test results. It also provides customizable test display names, making it easier to understand which test cases are failing. The following code shows it.
@Test
@DisplayName("Custom display name for this test")
void testWithCustomDisplayName() {
// Test code here
}
5. Parameterized Tests
Moreover, JUnit 5 simplifies parameterized testing by introducing the @ParameterizedTest
annotation. This allows you to write concise and readable parameterized tests without the need for complex test runners.
@ParameterizedTest
@ValueSource(ints = {1, 2, 3, 4, 5})
void testIsEven(int number) {
assertTrue(number % 2 == 0);
}
Conclusion
In conclusion, JUnit 5 brings a wealth of new features and enhancements to Java unit testing. Its modular architecture, improved annotations, and dynamic testing capabilities make it a powerful choice for developers. If you’re still using JUnit 4, it’s time to consider upgrading to JUnit 5 to take advantage of these features and improve your unit testing practices.
In upcoming posts, we’ll dive deeper into some of these features, exploring practical examples and best practices for using JUnit 5 effectively in your Java projects.
Stay tuned for more JUnit 5 tutorials and insights!
Next: Integration Testing with JUnit
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
100+ MCQs On Java Architecture
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
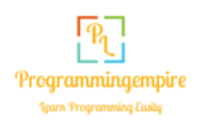