In this article, I will present some of the Hibernate Practical Problems and Their Solutions that will help you work with Hibernate.
Basically, Hibernate is a Java Framework for ORM (Object Relational Modeling). In other words, Hibernate provides a mapping of Java classes to the database tables.
The following list provides Hibernate Practical Problems and Their Solutions to get a basic understanding of Hibernate.
- To begin with, create a table Book in Oracle with the fields – book_title, author, book_id, and price. Also, create a corresponding JavaBean class. Further, insert a record in the Book table using the Hibernate mapping file (.hbm.xml).
- After that, change the Book table such that now the book_id is an auto-increment field. Now insert ten Book objects in this table using Hibernate.
- Further, insert some more records in the Book table. However, the values of the book_id field should be taken from a sequence. Create the sequence at the back end.
- Display the records from the Book table. Also, using a separate program display the book details where book_id is 7 and the book price is less than 500.
- Create an HTML form that asks for the book_id from the user and delete the Book object corresponding to that book_id. However, if that id doesn’t exist, display an error message.
- In another HTML form, accept the book_id and update in price. When the user clicks on the submit button, update the price corresponding to that book_id, if the book_id exists. Otherwise, display an error message.
- Demonstrate the use of the following annotations on the Book table in a hibernate program: @Entity, @Table, @Id, @Column.
- When do we use HQL? Write a Hibernate program to display the Book details using HQL.
- Now display only the book_title, and price of all book records using HQL.
- Similarly, display all the book_titles which start with ‘C’.
- Display the total number of book records.
- Now add another field named Category in the Book table. Display book records on the basis of Category.
Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
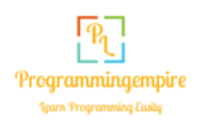