This blog provides 30 MCQs on Servlet Config and Servlet Context.
This set of 30 multiple-choice questions (MCQs) on Servlet Config and Servlet Context offers a comprehensive assessment of your knowledge related to the configuration and context management aspects of Java servlets. These questions cover critical concepts, such as servlet initialization, configuration parameters, and how servlets interact with their web application environment.
Servlet Config (Questions 1-15)
1. What is the purpose of the ServletConfig object in a Java Servlet?
a) To store servlet-specific initialization parameters
b) To store user session data
c) To manage HTTP requests and responses
d) To define servlet mapping rules
2. Which method is used to retrieve a ServletConfig object within a servlet?
a) getServletConfig()
b) getServletContext()
c) getInitParameter()
d) getConfigParameter()
3. In a servlet, which method is typically used to access initialization parameters from ServletConfig?
a) doGet()
b) doPost()
c) init()
d) service()
4. What is the purpose of the getInitParameter(String paramName) method in ServletConfig?
a) To retrieve the servlet’s context path
b) To retrieve the servlet’s name
c) To retrieve the value of an initialization parameter
d) To retrieve the servlet’s request URL
5. Servlet initialization parameters are typically defined in which configuration file?
a) web.xml
b) servlet.xml
c) index.html
d) application.properties
6. Which of the following statements is true about ServletConfig parameters?
a) They are set at runtime.
b) They can be modified by the servlet.
c) They are defined in the servlet code.
d) They are configured in the web server.
7. Which method is called by the servlet container to initialize a servlet?
a) init()
b) doGet()
c) doPost()
d) service()
8. Which of the following is NOT a valid way to retrieve a ServletConfig object within a servlet?
a) Using the getServletConfig() method
b) Using the this.getServletConfig() method
c) Using the getServletContext().getServletConfig() method
d) Using the getServletConfig().getServletContext() method
9. How can you access a ServletConfig parameter named “databaseURL” in a servlet?
a) config.getParameter(“databaseURL”)
b) this.getInitParameter(“databaseURL”)
c) config.getServletContext().getParameter(“databaseURL”)
d) context.getParameter(“databaseURL”)
10. Which of the following is true about ServletConfig parameters?
a) They are available only within the servlet’s doGet() method.
b) They are global parameters accessible by all servlets.
c) They can be changed at runtime by the servlet.
d) They are stored in the web.xml file.
11. How can you access the servlet’s name using ServletConfig?
a) this.getName()
b) config.getServletName()
c) config.getInitParameter(“servlet-name”)
d) getServletContext().getServletName()
12. In which method should you typically access ServletConfig parameters?
a) init()
b) doPost()
c) doGet()
d) service()
13. Which method is called only once during the lifecycle of a servlet to perform one-time initialization?
a) init()
b) doGet()
c) doPost()
d) service()
14. What happens if you try to access a non-existent initialization parameter using getInitParameter()?
a) It returns null.
b) It throws a ServletException.
c) It returns an empty string.
d) It returns a default value.
15. How can you access the ServletContext from within a ServletConfig object?
a) this.getServletContext()
b) config.getServletContext()
c) ServletConfig.getServletContext()
d) ServletContext.getServletConfig()
Servlet Context (Questions 16-30)
16. What is the purpose of the ServletContext object in a Java Servlet?
a) To store user session data
b) To manage HTTP requests and responses
c) To store servlet-specific initialization parameters
d) To provide information about the web application
17. How can you retrieve a ServletContext object within a servlet?
a) Using the getServletContext() method
b) Using the getServletConfig() method
c) Using the this.getServletContext() method
d) Using the config.getServletContext() method
18. ServletContext attributes are typically scoped to:
a) A single session
b) A single request
c) The entire web application
d) A single servlet
19. Which method is used to set an attribute in the ServletContext?
a) setAttribute(String name, Object value)
b) setContextAttribute(String name, Object value)
c) addAttribute(String name, Object value)
d) setApplicationAttribute(String name, Object value)
20. How can you retrieve a ServletContext attribute named “counter”?
a) context.getAttribute(“counter”)
b) this.getServletContext().getAttribute(“counter”)
c) config.getContextAttribute(“counter”)
d) ServletContext.getAttribute(“counter”)
21. ServletContext attributes are typically shared among:
a) All servlets in the application
b) All clients accessing the application
c) All sessions within the application
d) All requests within the application
22. Which of the following statements is true about ServletContext parameters?
a) They can be modified at runtime by the servlet.
b) They are stored in the web.xml file.
c) They are specific to individual servlets.
d) They are accessed using the getParameter() method.
23. How can you access the context path of a web application using ServletContext?
a) context.getPath()
b) context.getContextPath()
c) config.getServletContext().getPath()
d) ServletContext.getWebPath()
24. Which method is used to retrieve the MIME type of a file from the ServletContext?
a) getMimeType(String file)
b) getContentType(String file)
c) getServletContext().getMimeType(String file)
d) getMIMEType(String file)
25. How can you obtain a reference to the ServletContext from within a servlet?
a) this.getServletConfig().getServletContext()
b) this.getServletContext()
c) ServletConfig.getServletContext()
d) config.getServletContext()
26. Which of the following is NOT a valid way to set an attribute in the ServletContext?
a) context.setAttribute(“attributeName”, attributeValue)
b) context.putAttribute(“attributeName”, attributeValue)
c) this.getServletContext().setAttribute(“attributeName”, attributeValue)
d) getServletContext().setAttribute(“attributeName”, attributeValue)
27. What happens when you set a ServletContext attribute with the same name as an existing attribute?
a) The new attribute overwrites the existing one.
b) An exception is thrown.
c) Both attributes coexist with the same name.
d) The behavior is undefined.
28. Which of the following is NOT a valid use case for ServletContext attributes?
a) Storing database connections for reuse
b) Sharing data among multiple servlets
c) Storing user-specific session data
d) Caching application-wide configuration
29. In a servlet, how can you access the ServletContext’s initialization parameters?
a) context.getParameter(“paramName”)
b) config.getServletContext().getParameter(“paramName”)
c) getServletContext().getParameter(“paramName”)
d) config.getInitParameter(“paramName”)
30. ServletContext attributes are available for the entire lifecycle of:
a) A single servlet
b) A single request
c) A single session
d) The entire web application
Answers
1. a) To store servlet-specific initialization parameters
2. a) getServletConfig()
3. c) init()
4. c) To retrieve the value of an initialization parameter
5. a) web.xml
6. d) They are configured in the web server.
7. a) init()
8. c) Using the getServletContext().getServletConfig() method
9. b) this.getInitParameter(“databaseURL”)
10. d) They are stored in the web.xml file.
11. b) config.getServletName()
12. a) init()
13.a) init()
14. a) It returns null.
15. b) config.getServletContext()
16. d) To provide information about the web application
17. a) and c) Using the getServletContext() method
18. c) The entire web application
19. a) setAttribute(String name, Object value)
20. b) this.getServletContext().getAttribute(“counter”)
21. a) All servlets in the application
22. b) They are stored in the web.xml file.
23. b) context.getContextPath()
24. c) getServletContext().getMimeType(String file)
25. b) this.getServletContext()
26. b) context.putAttribute(“attributeName”, attributeValue)
27. a) The new attribute overwrites the existing one.
28. c) Storing user-specific session data
29. d) config.getInitParameter(“paramName”)
30. d) The entire web application
Further Reading
30 MCQs on Assert Method and Annotations in Java
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
100+ MCQs On Java Architecture
30 MCQs on Servlets – Get and Post Requests
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
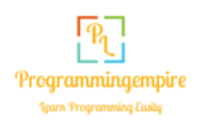