This article describes Mocking with JUnit.
Mocking with JUnit: Leveraging Mockito for Effective Testing
Testing is a crucial part of software development, and often, you’ll need to isolate components or dependencies to ensure that your tests are focused and reliable. This is where mocking comes into play. Basically, Mocking allows you to create simulated versions of objects or dependencies that your code interacts with. In the Java world, Mockito is a popular framework for achieving this. In this blog post, we’ll explore how to use Mockito effectively with JUnit to streamline your testing process.
What Is Mockito?
Mockito is a widely used mocking framework in the Java ecosystem. It enables you to create mock objects, stub method calls on these objects, and verify interactions with them. In fact, Mockito simplifies the testing of components that have dependencies by allowing you to replace those dependencies with mocks.
Setting Up Mockito
Before diving into the details of how to use Mockito with JUnit, you need to set up Mockito in your Java project. So, you can do this by adding the Mockito dependency to your build.gradle or pom.xml file if you’re using Gradle or Maven, respectively. The following example shows how to include Mockito in your Gradle build.
dependencies {
testImplementation 'org.mockito:mockito-core:3.11.2'
}
Once you’ve added the dependency, you’re ready to start using Mockito in your JUnit tests.
Creating Mock Objects
Let’s say you have a class UserService
that depends on a UserRepository
interface. In order to test UserService
, you want to create a mock UserRepository
to isolate it from the actual database. The following code shows how you can create a mock object using Mockito.
import static org.mockito.Mockito.*;
// Create a mock UserRepository
UserRepository userRepositoryMock = mock(UserRepository.class);
Therefore, with this mock object, you can define how it should behave when its methods are called during testing.
Stubbing Method Calls
Also, Mockito allows you to specify the behavior of mock objects by stubbing method calls. For example, you can make the mock UserRepository
return a predefined user when its findById
method is called. The following code shows this.
User expectedUser = new User("john.doe@example.com", "John Doe");
when(userRepositoryMock.findById(1L)).thenReturn(expectedUser);
This stubbing ensures that when findById
is called with the argument 1L
, it will return the expectedUser
object.
Verifying Interactions
In fact, one of the powerful features of Mockito is the ability to verify interactions with mock objects. So, you can check if specific methods were called and how many times they were invoked. For instance, to verify that the save
method on the mock UserRepository
was called once with a specific user object, you can do the following.
User userToSave = new User("jane.smith@example.com", "Jane Smith");
userService.saveUser(userToSave);
verify(userRepositoryMock, times(1)).save(userToSave);
Using Argument Matchers
Also, Mockito provides argument matchers to verify that a method was called with specific arguments without specifying the exact values. For example, to verify that the delete
method was called with any User
object, you can use an argument matcher like this.
userService.deleteUser(any(User.class));
verify(userRepositoryMock).delete(any(User.class));
Cleaning Up
After each test, it’s essential to clean up your mock objects to ensure they don’t interfere with subsequent tests. For this purpose, you can use the reset
method to reset the mock’s behavior.
reset(userRepositoryMock);
Conclusion
In conclusion, Mocking with JUnit and Mockito is a valuable skill for writing effective and focused unit tests. By creating mock objects, stubbing method calls, and verifying interactions, you can isolate components and dependencies in your code, leading to more reliable and maintainable tests. Mockito’s expressive API makes it a go-to choice for Java developers when it comes to mocking in testing. So, start using Mockito with JUnit, and you’ll be well on your way to improving the quality of your Java applications through thorough testing.
Happy testing!
Next: JUnit 5 Features and Upgrades
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
100+ MCQs On Java Architecture
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
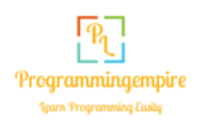