In this article, I will explain JUnit Test Suites.
JUnit Test Suites: Organizing and Running Your Tests
JUnit is a powerful Java testing framework that allows developers to write and run unit tests for their code. As your codebase grows, you’ll likely have multiple test classes covering various aspects of your application. In this blog post, we’ll explore how to organize and run these tests efficiently using JUnit test suites.
Why Use Test Suites?
Test suites in JUnit provide a way to group related test classes together. The following list shows the benefits we get by using a test suit.
- Organize Tests. When you have numerous test classes, it’s essential to keep them organized. In fact, test suites help you categorize tests based on their functionality.
- Run Specific Sets of Tests. Also, you may want to run only a subset of your tests during development or before a release. Test suites allow you to select and execute specific groups of tests.
- Improve Test Maintenance. When you make changes to your codebase, you might need to update some tests. With test suites, you can quickly identify which tests are affected and need modification.
Creating a JUnit Test Suite
Creating a test suite in JUnit is straightforward. In order to create a test suit, you can use the @RunWith
and @Suite.SuiteClasses
annotations to define a suite class. For example.
import org.junit.runner.RunWith;
import org.junit.runners.Suite;
@RunWith(Suite.class)
@Suite.SuiteClasses({
TestClass1.class,
TestClass2.class,
// Add more test classes here
})
public class MyTestSuite {
// This class can be empty
}
In the above example:
@RunWith(Suite.class)
indicates that this class is a test suite.@Suite.SuiteClasses
lists the test classes that should be included in the suite.
Also, you can add as many test classes as needed to the @SuiteClasses
annotation.
Running the Test Suite
In order to run the test suite, you can right-click on the suite class in your IDE and select “Run As -> JUnit Test.” Alternatively, you can run it programmatically using the JUnitCore
class. For example.
import org.junit.runner.JUnitCore;
import org.junit.runner.Result;
import org.junit.runner.notification.Failure;
public class TestSuiteRunner {
public static void main(String[] args) {
Result result = JUnitCore.runClasses(MyTestSuite.class);
for (Failure failure : result.getFailures()) {
System.out.println(failure.toString());
}
System.out.println("All tests passed: " + result.wasSuccessful());
}
}
Tips for Using Test Suites Effectively
- Keep Suites Focused. Create suites based on logical groupings of tests. For example, you might have separate suites for unit tests and integration tests.
- Update Suites as Needed. As your code evolves, remember to update your test suites to include new test classes or remove obsolete ones.
- Run Suites in Your CI/CD Pipeline. Integrate the execution of test suites into your continuous integration/continuous deployment (CI/CD) pipeline to ensure that tests are automatically run with every code change.
- Use Annotations and Naming Conventions. Adopt a naming convention for your test suite classes to make them easily recognizable, such as appending “Suite” to the test suite class name.
In conclusion, JUnit test suites are a valuable tool for organizing and running your tests efficiently. By following best practices and keeping your suites up to date, you can maintain a robust and reliable testing strategy for your Java projects.
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
100+ MCQs On Java Architecture
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
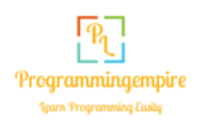