In this article, I will share Best Practices for Writing JUnit Tests.
Best Practices for Writing JUnit Tests
JUnit is a powerful testing framework in the Java ecosystem, widely used by developers to ensure the reliability and correctness of their code. While writing tests with JUnit is relatively straightforward, there are some best practices you should follow to create effective and maintainable test suites. In this blog post, we’ll explore these best practices step by step.
1. Clear and Descriptive Test Names
Start with a clear and descriptive name for your test methods. A good test name should convey the purpose of the test and what is being tested. This makes it easier to identify the problem when a test fails.
@Test
public void shouldCalculateTotalPrice() {
// ...
}
2. Use Arrange-Act-Assert (AAA) Pattern
Follow the AAA pattern in your test methods:
- Arrange: Set up the initial state and input for your test.
- Act: Invoke the method or code you want to test.
- Assert: Verify that the expected outcome matches the actual result.
@Test
public void shouldCalculateTotalPrice() {
// Arrange
ShoppingCart cart = new ShoppingCart();
cart.addItem(new Item("Product A", 10.0));
// Act
double totalPrice = cart.calculateTotalPrice();
// Assert
assertEquals(10.0, totalPrice, 0.01);
}
3. Avoid Global State and Side Effects
Tests should be isolated from each other. Avoid relying on global state or shared resources that might introduce side effects between tests. Each test should start with a clean slate.
4. Use Setup and Teardown Methods
JUnit provides @Before
and @After
annotations for setup and teardown tasks. Use them to initialize and clean up resources that are common to multiple test methods.
@Before
public void setUp() {
// Initialize common resources
}
@After
public void tearDown() {
// Clean up resources
}
5. Group Related Tests with Test Suites
If you have multiple test classes, you can group them into test suites using the @RunWith(Suite.class)
annotation. This helps organize and execute tests logically.
@RunWith(Suite.class)
@Suite.SuiteClasses({CalculatorTest.class, ShoppingCartTest.class})
public class TestSuite {
// This class can be empty
}
6. Parametrize Tests
JUnit allows you to create parameterized tests using the @ParameterizedTest
annotation. This is especially useful when you want to run the same test with different inputs.
@ParameterizedTest
@ValueSource(ints = {1, 2, 3})
public void shouldCheckIfNumberIsPositive(int number) {
assertTrue(number > 0);
}
7. Use Matchers for Complex Assertions
For complex data structures or objects, consider using Hamcrest matchers or custom matchers to create more expressive and readable assertions.
@Test
public void shouldContainItemWithName() {
List<Item> items = // ...
assertThat(items, hasItem(hasProperty("name", equalTo("Product A"))));
}
8. Regularly Review and Refactor Tests
Just like production code, tests can become messy over time. Regularly review and refactor your tests to keep them clean, maintainable, and up-to-date with your evolving codebase.
In conclusion, writing effective JUnit tests is crucial for ensuring the quality of your Java applications. By following these best practices, you can create tests that are not only reliable but also easy to understand and maintain. Happy testing!
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
100+ MCQs On Java Architecture
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
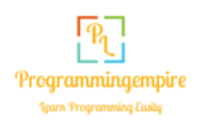