In this article, I will discuss JUnit and Code Coverage.
JUnit and Code Coverage: Ensuring Comprehensive Testing
When it comes to software testing, writing unit tests using JUnit is a fundamental practice for ensuring the reliability and stability of your code. However, writing tests is just one part of the equation. Understanding how much of your code is actually being tested is equally important. This is where code coverage comes into play. In this blog post, we will delve into the world of JUnit and code coverage to help you ensure comprehensive testing in your Java applications.
Understanding Code Coverage
Basically, Code coverage is a metric that measures the percentage of your source code that is executed during your tests. It provides valuable insights into how well your tests exercise your codebase. The goal is to have high code coverage, ideally close to 100%, to minimize the chances of undiscovered bugs and vulnerabilities.
Code coverage is typically categorized into three main types. For instance, we can measure statement coverage, branch coverage, and path coverage.
- Statement Coverage. This measures the percentage of code statements (lines) executed during tests. It’s the most basic form of coverage.
- Branch Coverage. Similarly, Branch coverage goes a step further by ensuring that all possible branches (if statements, loops, etc.) within your code are exercised by your tests.
- Path Coverage. Likewise, Path coverage is the most comprehensive form, aiming to test every possible path through your code. Achieving 100% path coverage can be incredibly challenging and may not always be practical.
Integrating Code Coverage with JUnit
In fact, JUnit itself doesn’t provide built-in code coverage tools. However, there are third-party libraries and plugins that seamlessly integrate with JUnit to measure code coverage. One of the most popular options is JaCoCo.
Setting Up JaCoCo
In order to get started with JaCoCo and JUnit, follow these steps.
- Add JaCoCo Maven or Gradle plugin. Depending on your build system, add the JaCoCo plugin to your project’s configuration.
- Configure JaCoCo. Then, customize your JaCoCo configuration to specify which classes and packages you want to measure coverage for.
- Run JUnit Tests with JaCoCo. After that, execute your JUnit tests with JaCoCo instrumentation enabled. This will track which lines and branches of your code are executed during the tests.
- Generate Reports. After running your tests, you can generate comprehensive HTML or XML reports using JaCoCo. These reports will visually display your code coverage.
Interpreting Code Coverage Reports
Once you’ve generated code coverage reports, it’s essential to understand how to interpret them.
- Coverage Percentage. This is the overall percentage of code coverage for your project. So, aim for high percentages, but keep in mind that 100% coverage may not always be realistic.
- Coverage by Class/Method. Reports usually break down coverage by class and method, making it easy to identify specific areas that need more attention.
- Uncovered Lines/Branches. Then, look for sections of your code with low or no coverage. These are potential areas where you should write additional tests.
Benefits of Code Coverage
Code coverage offers several benefits. For example.
- Bug Detection. Uncovered code is more likely to contain bugs. Therefore, code coverage helps you identify areas where your tests may be lacking.
- Quality Assurance. It ensures that your tests are comprehensive and that your codebase is thoroughly tested.
- Documentation. Also, coverage reports serve as documentation for your testing efforts, helping you and your team understand the extent of testing.
- Continuous Improvement. By regularly monitoring code coverage, you can continuously improve your test suite and ensure better software quality over time.
Conclusion
In conclusion, incorporating code coverage into your JUnit testing workflow is a vital step in maintaining software quality. Basically, it helps you identify untested code, reduce bugs, and build more reliable applications. Therefore, remember that code coverage is not an absolute guarantee of bug-free software, but it’s a crucial tool in your testing arsenal. So, start measuring and improving your code coverage today, and your codebase will thank you in the long run.
Next: Advanced JUnit Techniques
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
100+ MCQs On Java Architecture
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
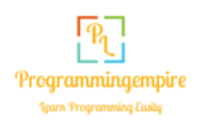