This article explores some Advanced JUnit Techniques.
Advanced JUnit Techniques: Rule-Based Testing, Custom Test Runners, and Custom Annotations
JUnit is a powerful Java testing framework that offers a wide range of features to help developers write effective and maintainable unit tests. In this blog post, we’ll explore some advanced JUnit techniques that go beyond the basics. Specifically, we’ll delve into rule-based testing, custom test runners, and custom annotations, showing you how to leverage these features to enhance your testing process.
Rule-Based Testing
Rule-based testing is a feature introduced in JUnit 4 that allows you to define custom test rules. These rules can be used to set up and tear down resources for your tests. They provide a way to encapsulate common test setup and teardown logic, making your test code cleaner and more readable.
Creating a Custom Test Rule
In order to create a custom test rule, you need to implement the TestRule
interface. This interface has a single method, apply(Statement base, Description description)
, which allows you to wrap test execution with custom logic. For example, you can create a rule to manage database connections or set up a temporary file system for your tests.
public class CustomTestRuleExample implements TestRule {
@Override
public Statement apply(Statement base, Description description) {
// Implement your custom logic here
return base; // Return the original statement to continue with the test
}
}
Using Custom Test Rules
Once you’ve created a custom test rule, you can apply it to your tests using the @Rule
annotation. This annotation makes your test class aware of the custom rule, and it will automatically execute the rule’s logic before and after each test method.
public class MyTest {
@Rule
public CustomTestRuleExample customRule = new CustomTestRuleExample();
@Test
public void myTest() {
// Your test logic here
}
}
Custom Test Runners
JUnit allows you to define custom test runners, which can change the way tests are executed. This is useful when you have specific requirements that cannot be achieved with the default JUnit behavior.
Creating a Custom Test Runner
To create a custom test runner, you need to extend the BlockJUnit4ClassRunner
class (for JUnit 4) or the JUnitPlatform
class (for JUnit 5). You can then override various methods to customize test execution, including test discovery, ordering, and execution.
public class CustomTestRunner extends BlockJUnit4ClassRunner {
public CustomTestRunner(Class<?> clazz) throws InitializationError {
super(clazz);
}
// Override methods to customize test execution
}
Using Custom Test Runners
In order to use your custom test runner, annotate your test class with @RunWith
(for JUnit 4) or use the @ExtendWith
annotation (for JUnit 5) to specify your custom runner.
@RunWith(CustomTestRunner.class) // For JUnit 4
public class MyCustomTest {
// Your test methods here
}
Custom Annotations
Custom annotations allow you to add metadata to your test methods or classes. You can create custom annotations to express specific conditions or behaviors that should be associated with your tests.
Creating a Custom Annotation
Creating a custom annotation is straightforward. Define the annotation using the @interface
keyword and specify any elements (attributes) that your annotation should have.
import java.lang.annotation.*;
@Retention(RetentionPolicy.RUNTIME)
@Target({ElementType.METHOD, ElementType.TYPE})
public @interface MyCustomAnnotation {
String value() default "";
// Add more attributes as needed
}
Using Custom Annotations
Once you’ve defined a custom annotation, you can use it to mark your test methods or classes. This allows you to associate custom behavior or conditions with your tests.
@MyCustomAnnotation("Some Custom Configuration")
public class MyTest {
@Test
public void myCustomTest() {
// Your test logic here
}
}
In conclusion, advanced JUnit techniques like rule-based testing, custom test runners, and custom annotations provide powerful ways to tailor your testing process to your specific needs. By mastering these advanced features, you can write more flexible and maintainable unit tests for your Java applications.
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
100+ MCQs On Java Architecture
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
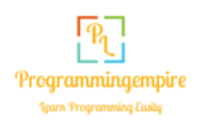