This article describes Jenkins Pipeline for JUnit Tests.
Jenkins Pipeline for JUnit Tests: A Step-by-Step Guide
Basically, Jenkins is a powerful automation server widely used for continuous integration and continuous delivery (CI/CD) pipelines. When it comes to automated testing in Java, JUnit is a go-to framework. Therefore, combining Jenkins and JUnit can streamline the testing process by automatically running tests whenever code changes are pushed to a repository. In this blog post, we will provide a detailed guide on setting up Jenkins pipelines for running JUnit tests automatically. In fact, it will help you ensure the quality of your Java applications with minimal manual effort.
Prerequisites:
Before we dive into creating a Jenkins pipeline for JUnit tests, make sure you have the following prerequisites in place.
- Jenkins Installed. Jenkins should be installed and running. You can download it from the official Jenkins website.
- Java Project. Also, you should have a Java project with JUnit tests already written. If not, create a simple Java project with some JUnit test cases.
- JUnit Dependencies. Ensure your project includes the necessary JUnit dependencies in its build system (e.g., Gradle or Maven).
Setting Up a Jenkins Pipeline for JUnit Tests:
Step 1: Install Required Jenkins Plugins
In order to create a Jenkins pipeline for running JUnit tests, you need the following Jenkins plugins.
- Pipeline. This plugin provides support for creating and managing Jenkins pipelines.
- JUnit. The JUnit plugin is essential for reporting and displaying test results.
So, install these plugins through the Jenkins plugin manager if they are not already installed.
Step 2: Create a New Pipeline Job
- In the Jenkins dashboard, click on “New Item” to create a new pipeline job.
- After that, provide a name for your job and select the “Pipeline” project type. Click “OK” to proceed.
Step 3: Configure Your Pipeline Script
Further, in the pipeline job configuration, scroll down to the “Pipeline” section and select the “Pipeline script from SCM” option. Choose your version control system (e.g., Git) and provide the repository URL.
Step 4: Define the Pipeline Script
Now, it’s time to define your Jenkins pipeline script. The following code shows a basic example.
pipeline {
agent any
stages {
stage('Checkout') {
steps {
// Checkout your source code from the repository
checkout scm
}
}
stage('Build and Test') {
steps {
// Build your Java project (e.g., with Gradle or Maven)
sh './gradlew build'
// Run JUnit tests and generate test reports
junit '**/build/test-results/test/*.xml'
}
}
}
post {
always {
// Archive JUnit test results for display in Jenkins
archiveArtifacts 'build/test-results/test/*.xml'
}
}
}
The following points are considered in this script.
- To begin with, we start by checking out the source code from the repository.
- After that, we build the Java project (you might need to adapt this based on your build system).
- Then, we use the JUnit plugin to run tests and generate test reports.
- Finally, we archive the test results for display in Jenkins.
Step 5: Save and Run the Pipeline
Finally, save your pipeline configuration, and then click “Build Now” to trigger the pipeline manually. Also, you can configure webhooks or triggers to automate this process when changes are pushed to your repository.
Step 6: Review Test Results
Once the pipeline run is complete, you can review the JUnit test results in the Jenkins job dashboard. Failed tests will be highlighted, and you can dive into specific test reports for more details.
In conclusion, setting up a Jenkins pipeline for running JUnit tests automatically can significantly improve your development workflow. Because it ensures that new code changes don’t introduce regressions. Hence, with this guide, you can get started with Jenkins and JUnit integration. Certainly, it will pave the way for more efficient and reliable Java application development.
Next: JUnit Test Reporting and Visualization
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
100+ MCQs On Java Architecture
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
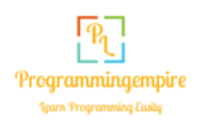