This blog describes How to Perform Various File Operations in Node.js.
The following code shows a basic Node.js application that performs various file operations like reading, writing, copying, and deleting files.
const fs = require('fs');
const path = require('path');
// Function to read a file
function readFile(filePath) {
fs.readFile(filePath, 'utf8', (err, data) => {
if (err) {
console.error('Error reading file:', err);
return;
}
console.log('File contents:', data);
});
}
// Function to write to a file
function writeFile(filePath, content) {
fs.writeFile(filePath, content, 'utf8', (err) => {
if (err) {
console.error('Error writing to file:', err);
return;
}
console.log('File written successfully');
});
}
// Function to copy a file
function copyFile(source, target) {
fs.copyFile(source, target, (err) => {
if (err) {
console.error('Error copying file:', err);
return;
}
console.log('File copied successfully');
});
}
// Function to delete a file
function deleteFile(filePath) {
fs.unlink(filePath, (err) => {
if (err) {
console.error('Error deleting file:', err);
return;
}
console.log('File deleted successfully');
});
}
// Usage examples
const sourceFile = path.join(__dirname, 'source.txt');
const targetFile = path.join(__dirname, 'target.txt');
readFile(sourceFile);
writeFile(targetFile, 'Hello, world!');
copyFile(sourceFile, targetFile);
deleteFile(targetFile);
source.txt
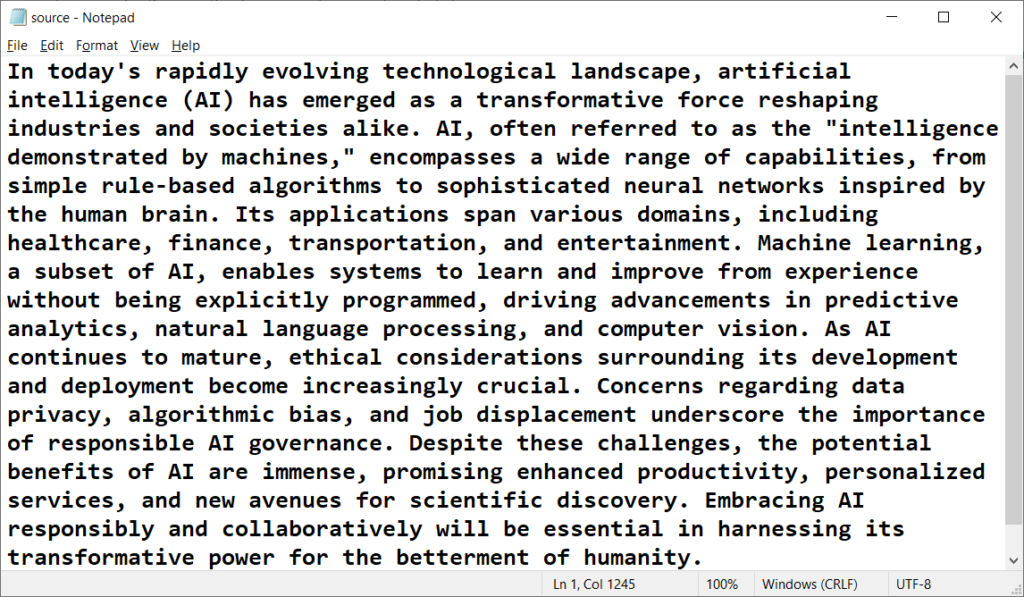
Output
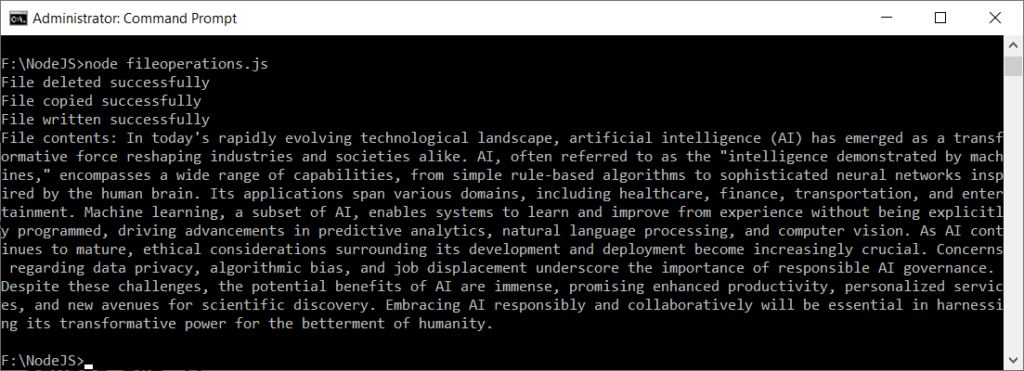
Make sure to create a source.txt
file in the same directory as this script to test the read, write, copy, and delete operations. This script uses the fs
(File System) module, which is built into Node.js, to perform file operations asynchronously.
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
100+ MCQs On Java Architecture
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
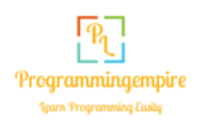