In this blog on Getting Started With NodeJS, I will explain how to start working with NodeJs using simple examples.
Node.js is a popular runtime for server-side JavaScript applications. It’s built on the V8 JavaScript runtime and allows you to run JavaScript code on the server side, enabling the development of scalable and efficient web applications. Here’s a step-by-step guide to getting started with Node.js:
Step 1: Install Node.js
Before you start using Node.js, you need to install it on your machine. You can download the latest version from the official website: Node.js Downloads (https://nodejs.org/en).
Installing Node.js

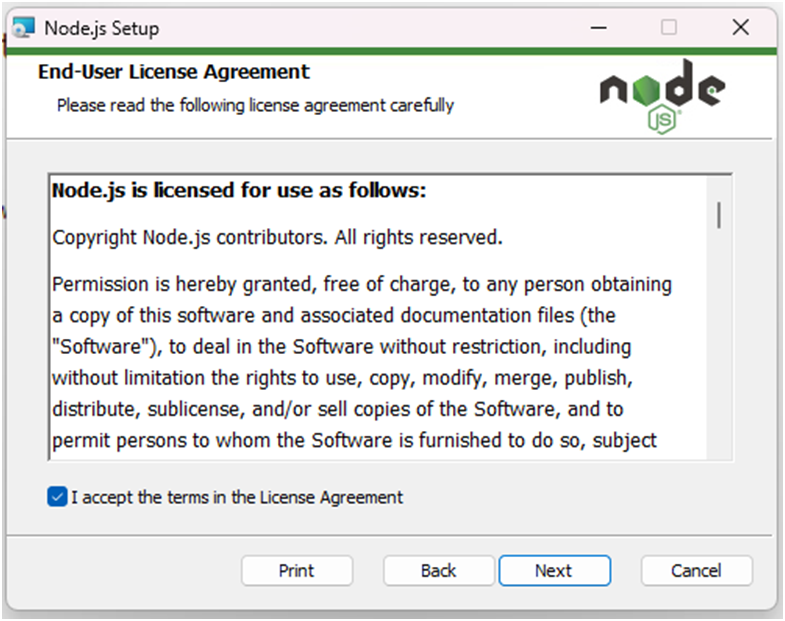

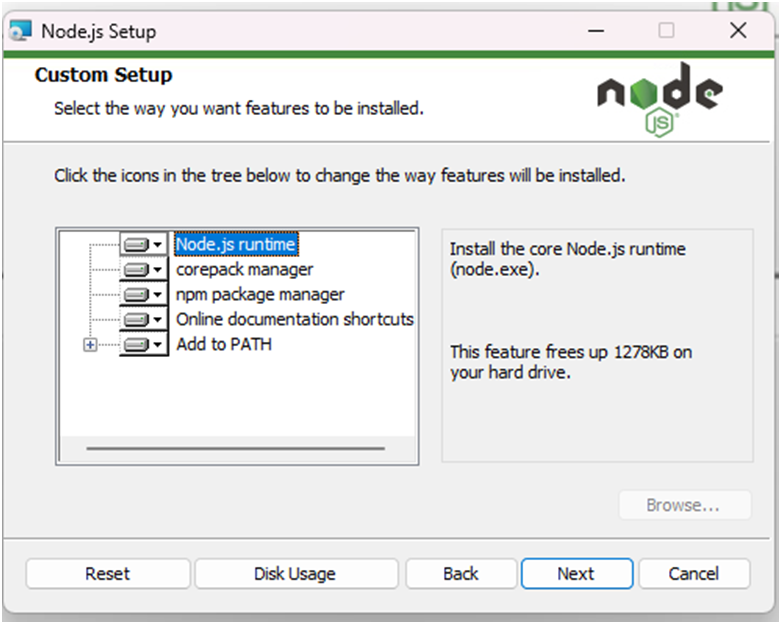
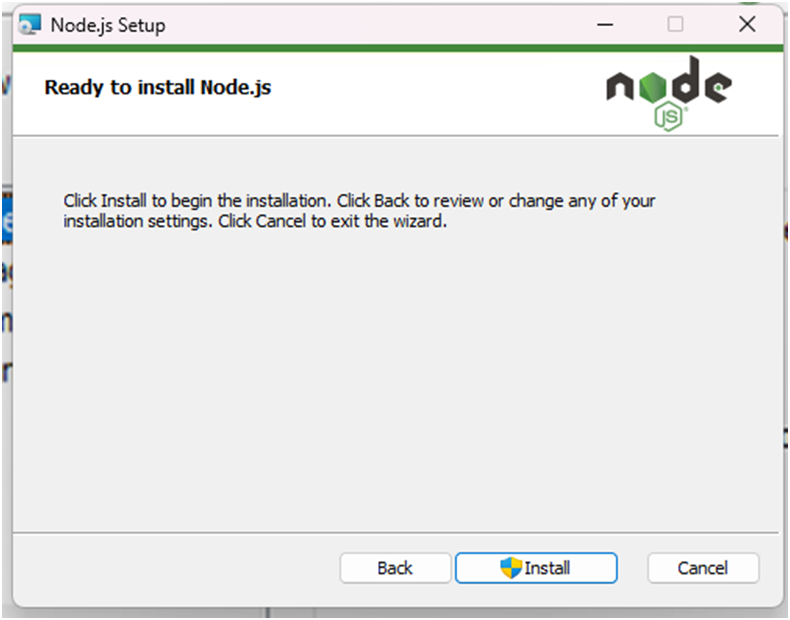
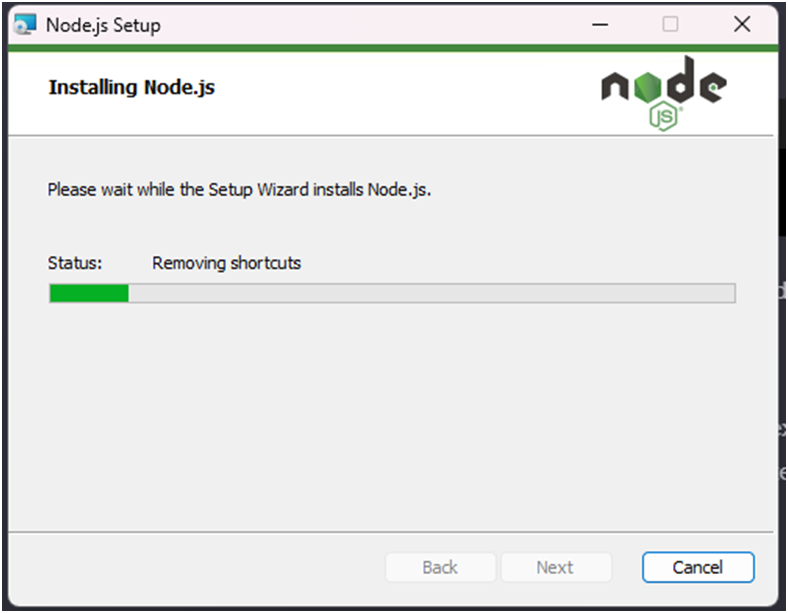
Step 2: Verify Installation
After installing Node.js, open a terminal or command prompt and run the following commands to verify that Node.js and npm (Node Package Manager) are installed:
node -v
npm –v
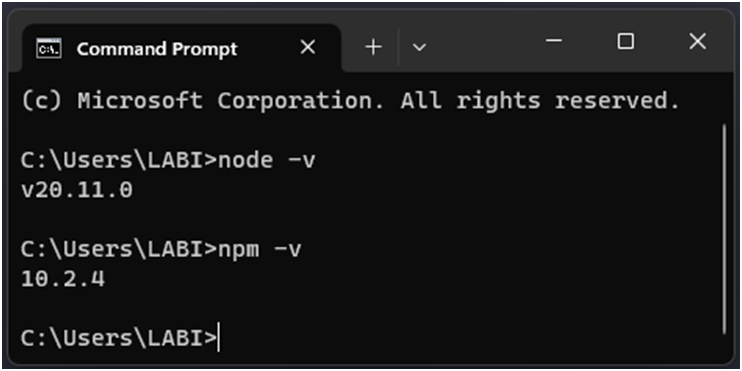
Step 3: Create a Simple Node.js Application
- Create a new folder for your project.
- Create a new file named app.js using a text editor of your choice (e.g., VSCode, Sublime Text, Atom):
// app.js
console.log('Hello, Node.js!');
Save the file and run it using the following command:
node app.js

Step 4: NPM (Node Package Manager)
NPM is the package manager for Node.js. It allows you to install and manage packages (libraries) for your Node.js projects.
Initialize a new package.json file for your project. Run the following command and follow the prompts:
npm init
Install a package. Let’s install the lodash library as an example:
npm install lodash

This command installs lodash and adds it to your package.json file as a dependency.
Step 5: Create a Web Server
Now, let’s create a simple web server using the Express framework. Install Express using npm:
Create a new file server.js:
// server.js
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('Hello, Express!');
});
app.listen(port, () => {
console.log(`Server is running at http://localhost:${port}`);
});
Run the server:
node server.js
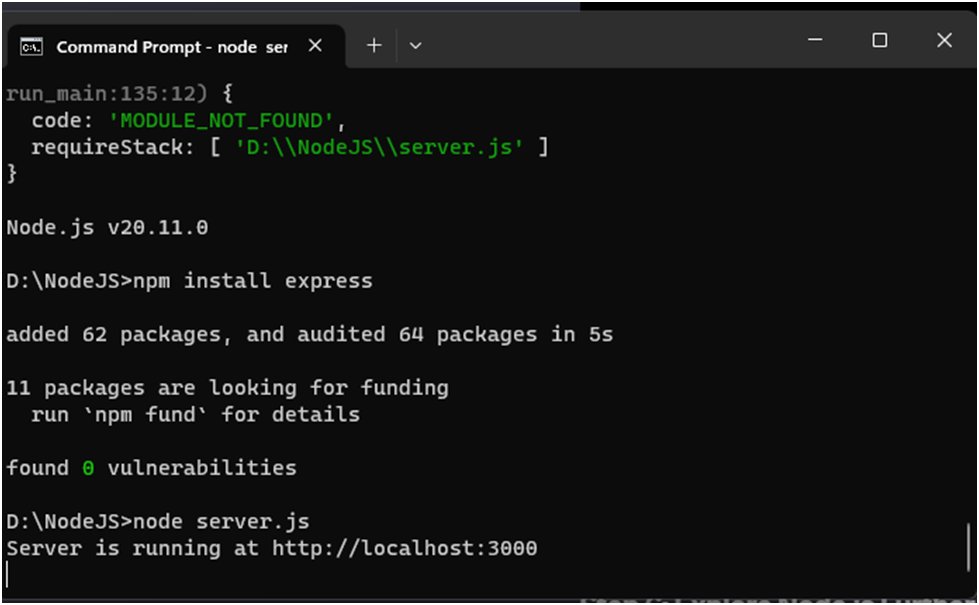

Further Reading
Understanding NodeJS With Examples
- Angular
- ASP.NET
- Bootstrap
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- NodeJS
- PHP
- Power Bi
- Python
- Scratch 3.0
- SQL
- TypeScript
- VB.NET
- Web Designing
- XML
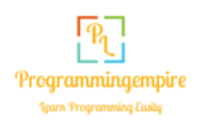