In this blog, I will explain How to Create a To Do List App Using React.
Introduction
The To-Do List App is a simple and interactive web application built using React.js, a popular JavaScript library for building user interfaces. This app provides users with a straightforward platform to manage their tasks, allowing them to add new to-do items, mark tasks as completed, and delete tasks they’ve accomplished. The application is designed with a clean and intuitive user interface, offering a seamless experience for organizing and tracking daily activities. By utilizing the principles of React.js, the To-Do List App demonstrates the power of component-based architecture and state management in creating dynamic and responsive user interfaces. Users can easily engage with their to-do list, making it a practical and accessible tool for maintaining productivity and staying organized. Whether for personal or professional use, this To-Do List App serves as an excellent starting point for those looking to explore React.js and its capabilities.
Requirements of How to Create a To Do List App Using React
- The app should display a list of to-do items.
- Further, users should be able to add new to-do items.
- Also, users should be able to mark a to-do item as completed.
- Finally, users should be able to delete a to-do item.
Steps
- Set up a new React.js project using Create React App or your preferred method.
- Create a component for the To-Do List (
TodoList
) that will render the list of to-do items. - Further, create a component for individual To-Do items (
TodoItem
). Each item should display its text and have buttons to mark it as completed and delete it. - Then, create a component for adding new to-do items (
AddTodo
). This component should have an input field and a button to add a new to-do item to the list. - Manage the state of the to-do list in a parent component (
App
). Pass down necessary props to child components. - Implement functionality to add new to-do items, mark items as completed, and delete items.
- Style your components to make the to-do list visually appealing.
Set up a new React App
Use Create React App to set up a new React project. Open your terminal and run.
npx create-react-app react-todo-app
cd react-todo-app
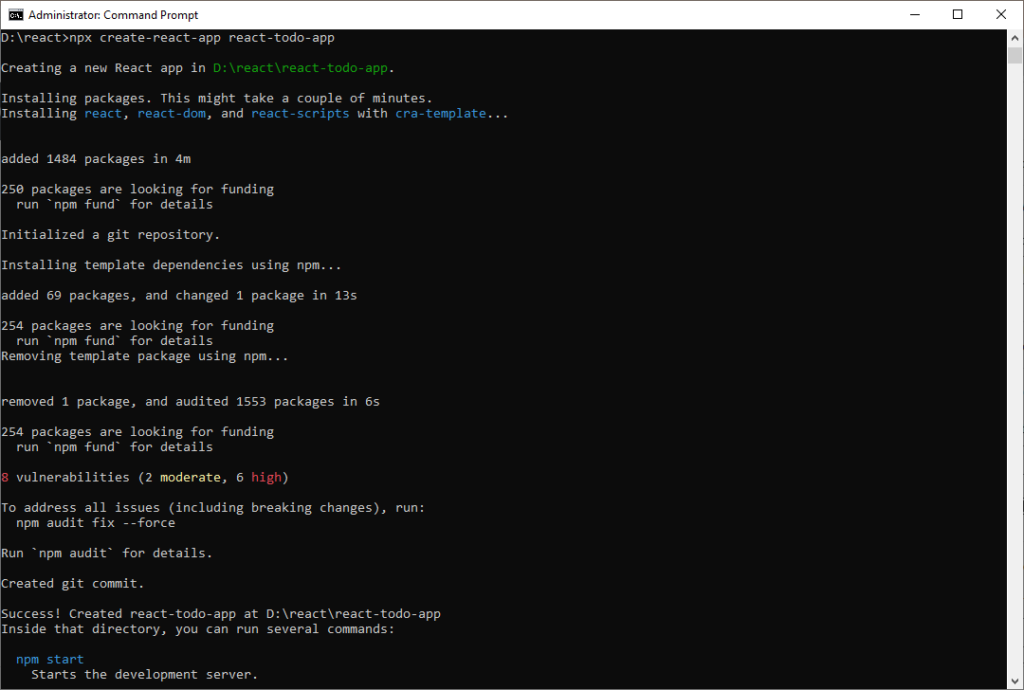
Create Components
After that, create the necessary components (App.js
, TodoList.js
, TodoItem.js
, AddTodo.js
) in the src
folder.
Example Component Structure
App.js
// src/App.js
import React, { useState } from 'react';
import TodoList from './TodoList';
import AddTodo from './AddTodo';
function App() {
const [todos, setTodos] = useState([]);
const addTodo = (text) => {
setTodos([...todos, { text, completed: false }]);
};
const toggleTodo = (index) => {
const newTodos = [...todos];
newTodos[index].completed = !newTodos[index].completed;
setTodos(newTodos);
};
const deleteTodo = (index) => {
const newTodos = [...todos];
newTodos.splice(index, 1);
setTodos(newTodos);
};
return (
<div>
<h1>To-Do List</h1>
<AddTodo addTodo={addTodo} />
<TodoList todos={todos} toggleTodo={toggleTodo} deleteTodo={deleteTodo} />
</div>
);
}
export default App;
TodoList.js
// src/TodoList.js
import React from 'react';
import TodoItem from './TodoItem';
function TodoList({ todos, toggleTodo, deleteTodo }) {
return (
<ul>
{todos.map((todo, index) => (
<TodoItem
key={index}
index={index}
todo={todo}
toggleTodo={toggleTodo}
deleteTodo={deleteTodo}
/>
))}
</ul>
);
}
export default TodoList;
TodoItem.js
// src/TodoItem.js
import React from 'react';
function TodoItem({ index, todo, toggleTodo, deleteTodo }) {
return (
<li>
<span style={{ textDecoration: todo.completed ? 'line-through' : 'none' }}>
{todo.text}
</span>
<button onClick={() => toggleTodo(index)}>Complete</button>
<button onClick={() => deleteTodo(index)}>Delete</button>
</li>
);
}
export default TodoItem;
AddTodo.js
// src/AddTodo.js
import React, { useState } from 'react';
function AddTodo({ addTodo }) {
const [text, setText] = useState('');
const handleAddTodo = () => {
if (text.trim() !== '') {
addTodo(text);
setText('');
}
};
return (
<div>
<input
type="text"
value={text}
onChange={(e) => setText(e.target.value)}
placeholder="Add a new to-do"
/>
<button onClick={handleAddTodo}>Add</button>
</div>
);
}
export default AddTodo;
Run the App
Back in the terminal, run the following command to start your React app:
npm start

Output
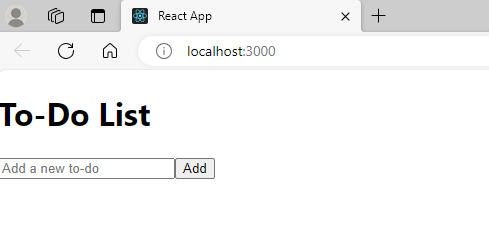
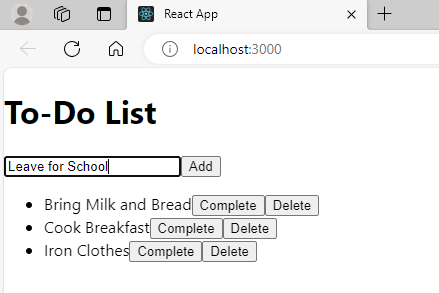

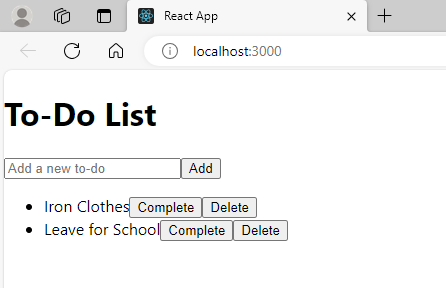
Further Reading
How to Create Class Components in React?
Examples of Array Functions in PHP
How to Create Functional Components in React?
Exploring PHP Arrays: Tips and Tricks
Registration Form Using PDO in PHP
Inserting Information from Multiple CheckBox Selection in a Database Table in PHP
PHP Projects for Undergraduate Students
Architectural Constraints of REST API
Creating a Classified Ads Application in PHP
How to Create a Bar Chart in ReactJS?
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
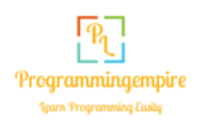