This blog provides 30+ Interview Questions on ReactJS along with the answers.
Here’s a list of over 30 interview questions on ReactJS that can help you prepare for a ReactJS interview:
General ReactJS Questions
- What is ReactJS, and why is it used?
- Explain the key features of React.
- What are the advantages of using React over other JavaScript frameworks?
- How does React differ from Angular and Vue.js?
- What is JSX in React? Why is it used?
- Describe the Virtual DOM and its role in React.
- What is the significance of Components in React?
- Differentiate between Functional Components and Class Components in React.
- How do you create a functional component in React?
- What is the purpose of
state
in React, and how is it initialized? - Explain the concept of props in React. How do you pass data from parent to child components?
- What are React Hooks, and how are they used?
- List some built-in hooks in React and describe their use cases.
- What is the significance of the
useState
hook in React? - How do you handle forms in React? Explain controlled and uncontrolled components.
- What is the React Context API, and why is it used?
- Describe the lifecycle methods of a Class Component in React.
- What is the useEffect hook in React, and why is it important?
- Explain the concept of routing in React. How can you implement routing?
- What is Redux, and how does it work with React?
- What are the advantages of using Redux in a React application?
- Describe the role of reducers and actions in Redux.
- What is the purpose of middleware in Redux?
- How can you optimize the performance of a React application?
- Explain the concept of code splitting in React.
Advanced ReactJS Questions
- What is the difference between React Fiber and the older reconciliation algorithm?
- Describe server-side rendering (SSR) in React. Why might you use SSR?
- What is lazy loading in React, and how can you implement it?
- Explain the concept of error boundaries in React.
- What is React.memo, and when should you use it?
- What are Higher-Order Components (HOCs) in React, and why might you use them?
- How do you manage state in a large-scale React application?
- What is the significance of PropTypes in React, and how do you use them?
- Describe the importance of key props in React lists.
- What is the purpose of the
shouldComponentUpdate
method in React? - Explain the concept of Portals in React and their use cases.
- What are the differences between React Native and React for web applications?
- Describe the concept of Render Props in React.
- How do you handle authentication in a React application?
- What is the role of Redux Thunk and Redux Saga in handling asynchronous actions?
- What is the purpose of the
react-router-dom
library, and how do you use it for routing in React applications?
Remember to prepare not just the answers but also practical examples and code snippets to showcase your understanding of ReactJS during the interview. React is a popular library, and interviewers often ask both theoretical and practical questions to assess your skills and knowledge.
Further Reading
Examples of Array Functions in PHP
Exploring PHP Arrays: Tips and Tricks
Registration Form Using PDO in PHP
Inserting Information from Multiple CheckBox Selection in a Database Table in PHP
PHP Projects for Undergraduate Students
Architectural Constraints of REST API
Creating a Classified Ads Application in PHP
How to Create a Bar Chart in ReactJS?
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
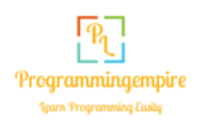