This blog describes how to toggle the visibility of content using state in React.
To toggle the visibility of content using state in React, you can use the useState
hook to manage the visibility state of the content. Here’s how you can do it.
ToggleContent.js
import React, { useState } from 'react';
const ToggleContent = () => {
// State variable to track the visibility
const [isVisible, setIsVisible] = useState(false);
// Function to toggle the visibility
const toggleVisibility = () => {
setIsVisible(!isVisible);
};
return (
<div>
<button onClick={toggleVisibility}>
{isVisible ? 'Hide Content' : 'Show Content'}
</button>
{isVisible && <div>This content is now visible!</div>}
</div>
);
};
export default ToggleContent;
App.js
In this example:
- We define a functional component called
ToggleContent
. - We use the
useState
hook to create a state variableisVisible
initialized tofalse
. This variable tracks the visibility state of the content. - We define a function
toggleVisibility
that toggles the value ofisVisible
when called. - We render a button that triggers the
toggleVisibility
function when clicked. The text of the button changes based on the current value ofisVisible
. - We conditionally render the content using
{isVisible && <div>This content is now visible!</div>}
. IfisVisible
istrue
, the content is rendered; otherwise, it’s not rendered.
Now, when you click the button, it will toggle the visibility of the content. If the content is visible, clicking the button will hide it, and vice versa.
Output

Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
100+ MCQs On Java Architecture
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
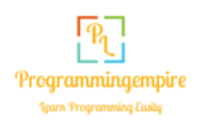