This blog describes how to change the background color of a component on button click using state in React.
You can change the background color of a component on button click using state in React by updating the state variable representing the background color when the button is clicked. Here’s how you can do it.
ColorChanger.js
import React, { useState } from 'react';
const ColorChanger = () => {
// State variable to track the background color
const [backgroundColor, setBackgroundColor] = useState('white');
// Function to change the background color
const changeColor = () => {
// Generate a random color (you can use any method to get a color)
const randomColor = '#' + Math.floor(Math.random()*16777215).toString(16);
setBackgroundColor(randomColor);
};
return (
<div style={{ backgroundColor: backgroundColor, padding: '20px' }}>
<button onClick={changeColor}>Change Color</button>
</div>
);
};
export default ColorChanger;
App.js
import React from 'react';
import ReactDOM from 'react-dom';
import ColorChanger from './ColorChanger';
const App = () => {
return (
<div>
<ColorChanger />
</div>
);
};
ReactDOM.render(
<React.StrictMode>
<App />
</React.StrictMode>,
document.getElementById('root')
);
export default App;
In this example:
- We define a functional component called
ColorChanger
. - We use the
useState
hook to create a state variablebackgroundColor
initialized to'white'
. This variable tracks the background color of the component. - We define a function
changeColor
that generates a random color and updates thebackgroundColor
state variable with the generated color when called. - We render a
<div>
element with the background color set to the value ofbackgroundColor
. We also include a button that triggers thechangeColor
function when clicked. - The inline style
{ backgroundColor: backgroundColor, padding: '20px' }
dynamically sets the background color of the<div>
based on the value of thebackgroundColor
state variable.
Now, when you click the button, it will change the background color of the component to a random color.
Output

Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
100+ MCQs On Java Architecture
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
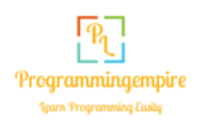