Here we provide 30 MCQs on JUnit along with their answers.
- What is JUnit?
a) A Java IDE
b) A Java unit testing framework
c) A Java build tool
d) A Java web framework
Correct Answer: b) A Java unit testing framework
- Which annotation is used to mark a method as a JUnit test method?
a) @Test
b) @TestMethod
c) @JUnitTest
d) @UnitTest
Correct Answer: a) @Test
- In JUnit, which assertion method is used to check if two objects are equal?
a) assertEquals()
b) assertEqual()
c) assertSame()
d) assertNotEquals()
Correct Answer: a) assertEquals()
- What does JUnit provide for organizing and executing test cases?
a) Test classes
b) Test runners
c) Test suites
d) All of the above
Correct Answer: d) All of the above
- Which JUnit assertion method is used to check if a condition is true?
a) assertTrue()
b) assertFalse()
c) assertCondition()
d) assertTruth()
Correct Answer: a) assertTrue()
- What is the purpose of the @Before annotation in JUnit?
a) It marks a method to run before every test method.
b) It marks a method to run after every test method.
c) It marks a method to run before the entire test class.
d) It marks a method to run after the entire test class.
Correct Answer: a) It marks a method to run before every test method.
- Which JUnit annotation is used to specify that a test method is expected to throw a particular exception?
a) @ExpectException
b) @ThrowsException
c) @Test(expected = Exception.class)
d) @ExpectedException
Correct Answer: c) @Test(expected = Exception.class)
- What is the purpose of the @After annotation in JUnit?
a) It marks a method to run before every test method.
b) It marks a method to run after every test method.
c) It marks a method to run before the entire test class.
d) It marks a method to run after the entire test class.
Correct Answer: b) It marks a method to run after every test method.
- Which JUnit annotation is used to disable a test method temporarily?
a) @Ignore
b) @Disable
c) @Exclude
d) @Skip
Correct Answer: a) @Ignore
- Which JUnit version introduced the parameterized tests feature?
a) JUnit 3
b) JUnit 4
c) JUnit 5
d) JUnit 6
Correct Answer: b) JUnit 4
11. What is the purpose of the @RunWith annotation in JUnit?
a) It specifies the test runner to be used for a test class.
b) It marks a method to run with a specific configuration.
c) It defines the order in which test methods are executed.
d) It sets up test dependencies.
Correct Answer: a) It specifies the test runner to be used for a test class.
12. In JUnit, what is the role of a test runner?
a) It defines test cases.
b) It executes test methods.
c) It generates test reports.
d) It generates code coverage reports.
Correct Answer: b) It executes test methods.
13. Which JUnit assertion method is used to check if two objects refer to the same object?
a) assertSame()
b) assertEquals()
c) assertNotSame()
d) assertNotEquals()
Correct Answer: a) assertSame()
14. In JUnit, which annotation is used to specify a timeout for a test method?
a) @Timeout
b) @Test(timeout = 1000)
c) @Test(1000)
d) @Test(timeout: 1000)
Correct Answer: b) @Test(timeout = 1000)
15. What is the purpose of JUnit’s TestSuite class?
a) It represents a single test case.
b) It groups multiple test classes into a suite.
c) It provides a way to configure test environments.
d) It is used to define custom assertions.
Correct Answer: b) It groups multiple test classes into a suite.
16. Which JUnit 5 extension provides support for parameterized tests?
a) @ParameterizedTest
b) @RunWith(Parameterized.class)
c) @Parameterized
d) @ParamTest
Correct Answer: a) @ParameterizedTest
17. In JUnit, which annotation is used to disable a specific test method conditionally?
a) @Disabled
b) @Ignore
c) @Test(disabled = true)
d) @IgnoreIf
Correct Answer: d) @IgnoreIf
18. What is the purpose of the @BeforeEach annotation in JUnit 5?
a) It marks a method to run before every test method.
b) It marks a method to run after every test method.
c) It marks a method to run before the entire test class.
d) It marks a method to run after the entire test class.
Correct Answer: a) It marks a method to run before every test method.
19. Which JUnit 5 annotation is used to group multiple test methods?
a) @TestGroup
b) @TestSuite
c) @TestMethodGroup
d) @TestFactory
Correct Answer: b) @TestSuite
20. What is the default access modifier for test methods in JUnit?
a) public
b) private
c) protected
d) package-private
Correct Answer: a) public
21. Which JUnit 5 annotation is used to specify the order in which test methods are executed within a test class?
a) @TestOrder
b) @TestMethodOrder
c) @TestSequence
d) @TestPriority
Correct Answer: b) @TestMethodOrder
22. In JUnit 5, what is the purpose of the @RepeatedTest annotation?
a) It marks a test method as a parameterized test.
b) It repeats a test method a specified number of times.
c) It marks a test method as disabled.
d) It groups test methods together.
Correct Answer: b) It repeats a test method a specified number of times.
23. Which JUnit 5 extension is used for dependency injection in test classes?
a) @Inject
b) @Autowired
c) @TestDependency
d) @ExtendWith
Correct Answer: d) @ExtendWith
24. What is the purpose of the @Tag annotation in JUnit 5?
a) It specifies the test runner to be used.
b) It assigns tags to test methods or classes.
c) It defines the execution order of test methods.
d) It sets up test dependencies.
Correct Answer: b) It assigns tags to test methods or classes.
25. In JUnit 5, which annotation is used to assert that an exception is thrown during a test?
a) @ExpectException
b) @AssertThrows
c) @Test(expected = Exception.class)
d) @ExpectedException
Correct Answer: b) @AssertThrows
26. Which JUnit 5 annotation is used to disable a test conditionally based on a system property?
a) @ConditionalOnProperty
b) @TestIfProperty
c) @Test(enabled = System.getProperty(“myProperty”) != null)
d) @DisabledIfSystemProperty
Correct Answer: d) @DisabledIfSystemProperty
27. What is the purpose of the @TestFactory annotation in JUnit 5?
a) It marks a factory method that produces test instances.
b) It specifies the factory class for creating test objects.
c) It defines a factory pattern for generating test data.
d) It marks a test method as a factory for other test methods.
Correct Answer: a) It marks a factory method that produces test instances.
28. Which JUnit 5 annotation is used to enable parameterized tests with dynamic test generation?
a) @DynamicTest
b) @ParameterizedTest
c) @TestFactory
d) @ParameterizedFactory
Correct Answer: a) @DynamicTest
29. In JUnit 5, what is the purpose of the @Timeout annotation?
a) It specifies the maximum execution time for a test method.
b) It marks a test method as a timeout test.
c) It sets a timeout for test class initialization.
d) It defines a timeout for test case reporting.
Correct Answer: a) It specifies the maximum execution time for a test method.
30. Which JUnit 5 annotation is used to specify test dependencies and control the order of test execution?
a) @TestDependency
b) @TestMethodOrder
c) @DependsOn
d) @ExecutionOrder
Correct Answer: c) @DependsOn
Further Reading
30 MCQs on Assert Method and Annotations in Java
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
100+ MCQs On Java Architecture
30 MCQs on Introduction to Servlets in Java
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
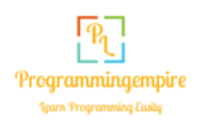