This blog describes how to render a list of items using the map function in React.
Rendering a list of items in React using the map
function is a common pattern. You typically create an array of JSX elements by mapping over your data array and then render this array within your component’s return statement. Here’s how you can do it:
Suppose you have an array of items like this.
const items = [
{ id: 1, name: 'Item 1' },
{ id: 2, name: 'Item 2' },
{ id: 3, name: 'Item 3' },
];
And you want to render each item in a list.
ItemList.js
import React from 'react';
const ItemList = ({ items }) => {
return (
<div>
<h2>Items</h2>
<ul>
{items.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
</div>
);
};
export default ItemList;
In this example:
- We define a functional component called
ItemList
. - We receive the
items
array as a prop. - Inside the component’s return statement, we use the
map
function to iterate over theitems
array. For each item, we return a<li>
element with the item’sname
as its content. - We include a
key
prop for each<li>
element. It’s important to provide a unique key for each item when rendering a list in React. Here, we’re using the item’sid
as the key. - Finally, we render the list within a
<ul>
element.
Now, you can use the ItemList
component and pass your array of items as props:
App.js
import React from 'react';
import ItemList from './ItemList';
const App = () => {
// Define the items array
const items = [
{ id: 1, name: 'Item 1' },
{ id: 2, name: 'Item 2' },
{ id: 3, name: 'Item 3' },
];
return (
<div>
{/* Pass the items array as a prop to the ItemList component */}
<ItemList items={items} />
</div>
);
};
export default App;
Output
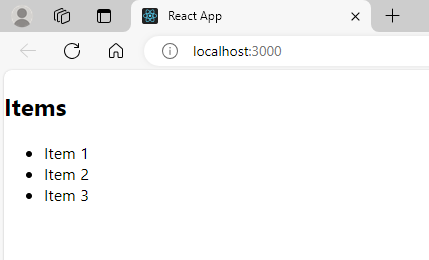
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
100+ MCQs On Java Architecture
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
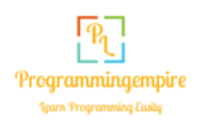