This blog describes how to create a counter app using functional components in React.
To create a counter app using functional components in React, you’ll need to maintain the state of the counter using the useState
hook. For example.
CounterApp.js
import React, { useState } from 'react';
const CounterApp = () => {
// State variable count and function setCount to update it
const [count, setCount] = useState(0);
// Function to increment the count
const increment = () => {
setCount(count + 1);
};
// Function to decrement the count
const decrement = () => {
setCount(count - 1);
};
return (
<div>
<h1>Counter App</h1>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
};
export default CounterApp;
In this example:
- We import React and the
useState
hook from ‘react’. - We define a functional component called
CounterApp
. - Inside the
CounterApp
component, we use theuseState
hook to create a state variablecount
initialized to 0, and a functionsetCount
to update its value. - We define two functions
increment
anddecrement
to handle the increment and decrement of thecount
state respectively. Inside these functions, we usesetCount
to update thecount
state. - We render the
count
state inside a<p>
element to display the current count value. - We render two buttons, one for incrementing and one for decrementing the count. We attach the
increment
anddecrement
functions to theonClick
event of these buttons.
This creates a simple counter app where you can increment and decrement the count. Each time you click the buttons, the count state updates, and the component re-renders to reflect the new count value.
App.js
import React from 'react';
import ReactDOM from 'react-dom';
import CounterApp from './CounterApp';
const App = () => {
return (
<div>
<CounterApp />
</div>
);
};
ReactDOM.render(
<React.StrictMode>
<App />
</React.StrictMode>,
document.getElementById('root')
);
export default App;
Output

Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
100+ MCQs On Java Architecture
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
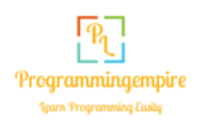