In this blog on How to Work With React JS, I will explain React JS using 5 simple programs.
React.js is a JavaScript library commonly used for building user interfaces. Here are five basic programs that demonstrate various aspects of React:
Hello World
- The simplest React program that renders a “Hello, World!” message.
// index.js
import React from 'react';
import ReactDOM from 'react-dom';
const App = () => {
return <div>Hello, World!</div>;
};
ReactDOM.render(<App />, document.getElementById('root'));
State and Event Handling
- A program that demonstrates the use of state and event handling in React.
// App.js
import React, { useState } from 'react';
const App = () => {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
};
export default App;
// index.js
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
ReactDOM.render(<App />, document.getElementById('root'));
Props
- A simple program that uses props to pass data between components.
// ParentComponent.js
import React from 'react';
import ChildComponent from './ChildComponent';
const ParentComponent = () => {
const message = 'Hello from Parent!';
return <ChildComponent message={message} />;
};
export default ParentComponent;
// ChildComponent.js
import React from 'react';
const ChildComponent = ({ message }) => {
return <div>{message}</div>;
};
export default ChildComponent;
Lists and Keys
- A program that demonstrates rendering a list of items using the
map
function and assigning keys to each element.
// ListComponent.js
import React from 'react';
const ListComponent = () => {
const items = ['Item 1', 'Item 2', 'Item 3'];
return (
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
);
};
export default ListComponent;
Conditional Rendering
- A program that shows how to conditionally render content based on a variable.
// ConditionalRendering.js
import React, { useState } from 'react';
const ConditionalRendering = () => {
const [isLoggedIn, setIsLoggedIn] = useState(false);
return (
<div>
{isLoggedIn ? (
<p>Welcome, User!</p>
) : (
<button onClick={() => setIsLoggedIn(true)}>Log In</button>
)}
</div>
);
};
export default ConditionalRendering;
Remember to set up a React development environment with tools like Create React App or configure your project to use React and ReactDOM for these examples to work.
Further Reading
Examples of Array Functions in PHP
How to Create a To Do List App Using React?
Exploring PHP Arrays: Tips and Tricks
Registration Form Using PDO in PHP
Inserting Information from Multiple CheckBox Selection in a Database Table in PHP
PHP Projects for Undergraduate Students
Architectural Constraints of REST API
Creating a Classified Ads Application in PHP
How to Create a Bar Chart in ReactJS?
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
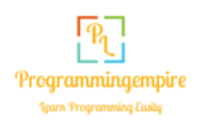