In this blog, I will discuss How to Create Functional Components in React.
Creating function components in React is a common and modern approach. Functional components are simpler and more concise than class components, especially with the introduction of React Hooks. Here’s a basic example of how to create a functional component:
Basic Example Demonstrating How to Create Functional Components in React
Here’s a basic example of how to create a functional component in React:
import React, { useState } from 'react';
const MyFunctionComponent = () => {
// State example using the useState hook
const [count, setCount] = useState(0);
// JSX that defines the component's UI
return (
<div>
<h1>Hello, I'm a function component!</h1>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increase Count</button>
</div>
);
};
export default MyFunctionComponent;
In this example:
- We import
React
and theuseState
hook from the ‘react’ module. - We define a function component named
MyFunctionComponent
using the arrow function syntax. - Inside the component, we use the
useState
hook to create a state variablecount
and a functionsetCount
to update its value. The initial value ofcount
is set to0
. - The component’s UI is defined using JSX, including an
<h1>
element and a<button>
element. Thecount
state is displayed, and clicking the button increases the count. - The component is exported as the default export of the module.
Functional components can also utilize other hooks, such as useEffect
for handling side effects, useContext
for accessing context values, and more.
Keep in mind that if you need to manage local component state or lifecycle methods, you can achieve this with the help of hooks. If you’re working on a project using the latest versions of React, functional components with hooks are the recommended way to build components.
Another Example
ChangeMyPet.js
import React, { useState } from 'react';
const ChangeMyPet = () => {
const [petData, setPetData] = useState({
flag: 0,
name: 'Cherry',
age: 1.5,
hair: 'Golden',
breed: 'Spitz',
});
const handleChangePet = () => {
setPetData((prevState) => ({
name: prevState.flag === 0 ? 'Charlie' : 'Cherry',
hair: prevState.flag === 0 ? 'White' : 'Golden',
flag: prevState.flag === 0 ? 1 : 0,
age: prevState.age, // Maintain other properties
breed: prevState.breed, // Maintain other properties
}));
};
return (
<div>
<h1>
Name: {petData.name}, Age: {petData.age}, Hair Color: {petData.hair}, Breed: {petData.breed}
</h1>
<button onClick={handleChangePet}>Change Pet</button>
</div>
);
};
export default ChangeMyPet;
App.js
// App.js
import React from 'react';
import ChangeMyPet from './ChangeMyPet';
function App() {
return (
<div className="App">
<ChangeMyPet />
</div>
);
}
export default App;
Output
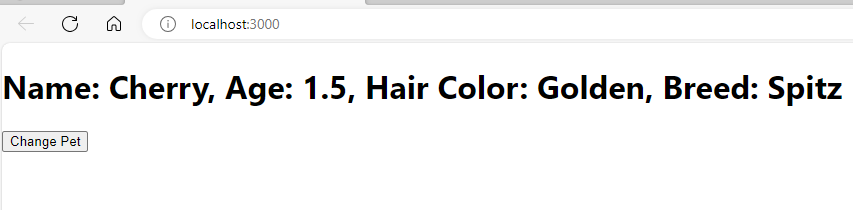
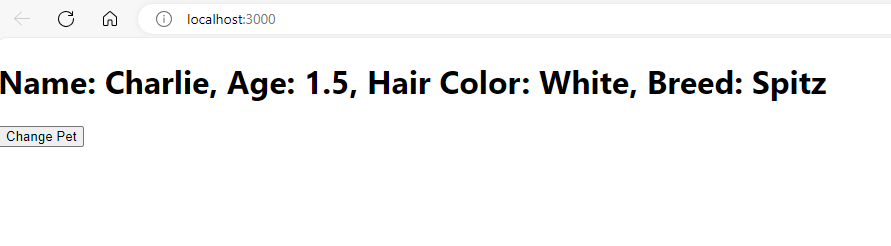
Import Statements
import React, { useState } from 'react';
The code imports the React
object and the useState
hook from the ‘react’ module. The useState
hook is a React Hook that allows functional components to manage state.
Function Component Declaration
const ChangeMyPet = () => {
This line declares a functional component named ChangeMyPet
using the arrow function syntax. Functional components are a modern and concise way to define components in React.
State Initialization
const [petData, setPetData] = useState({
flag: 0,
name: 'Cherry',
age: 1.5,
hair: 'Golden',
breed: 'Spitz',
});
The useState
hook is used to create a state variable petData
and its associated updater function setPetData
. The initial state is an object with properties such as flag
, name
, age
, hair
, and breed
.
handleChangePet Function
const handleChangePet = () => {
setPetData((prevState) => ({
name: prevState.flag === 0 ? 'Charlie' : 'Cherry',
hair: prevState.flag === 0 ? 'White' : 'Golden',
flag: prevState.flag === 0 ? 1 : 0,
age: prevState.age, // Maintain other properties
breed: prevState.breed, // Maintain other properties
}));
};
This function is called when the “Change Pet” button is clicked. It uses the setPetData
function with a callback that takes the previous state (prevState
) and returns a new state object. The logic inside the callback toggles the values of name
, hair
, and flag
based on the current value of flag
.
Render Method
return (
<div>
<h1>
Name: {petData.name}, Age: {petData.age}, Hair Color: {petData.hair}, Breed: {petData.breed}
</h1>
<button onClick={handleChangePet}>Change Pet</button>
</div>
);
The return
statement contains JSX that defines the structure of the component’s UI. It displays the current values of name
, age
, hair
, and breed
from the petData
state. A button is rendered, and its onClick
event is associated with the handleChangePet
function.
Export Statement
export default ChangeMyPet;
Finally, the ChangeMyPet
component is exported as the default export of this module, making it available for use in other parts of your application.
In summary, this functional component represents a pet with various attributes, and clicking the “Change Pet” button toggles between two sets of values for the pet’s name, hair color, and a flag indicating the change, all managed using the useState
hook.
Further Reading
How to Create Class Components in React?
Examples of Array Functions in PHP
Exploring PHP Arrays: Tips and Tricks
Registration Form Using PDO in PHP
Inserting Information from Multiple CheckBox Selection in a Database Table in PHP
PHP Projects for Undergraduate Students
Architectural Constraints of REST API
Creating a Classified Ads Application in PHP
How to Create a Bar Chart in ReactJS?
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
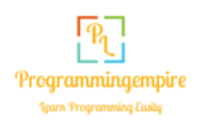