Today we will discuss some JUnit Best Practices for Android Development.
Some Important JUnit Best Practices for Android Development
Android app development is a dynamic field where quality assurance is crucial. JUnit, the popular Java testing framework, plays a significant role in ensuring the reliability and stability of your Android applications. In this blog post, we’ll explore some best practices for using JUnit effectively in the context of Android development.
1. Use AndroidJUnitRunner
When setting up your Android project for testing, it’s essential to use the AndroidJUnitRunner
as the test runner. This runner is optimized for Android and provides access to the Android Instrumentation framework, which allows you to interact with the Android system during testing. In order to configure it, add the following line to your build.gradle.
android {
defaultConfig {
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
}
2. Test Isolation
Also, ensure that your tests are isolated from each other and from the production code. While each test should run independently and not rely on the state set by other tests. In order to achieve this, use the @Before
and @After
annotations to set up and tear down test fixtures.
3. Arrange, Act, Assert (AAA) Pattern
Follow the Arrange, Act, Assert (AAA) pattern in your test methods. This pattern involves three distinct sections.
- Arrange: Set up the initial conditions for your test.
- Act: Perform the action or operation you want to test.
- Assert: Verify the expected outcomes or results.
This structure makes your tests more readable and helps pinpoint issues when they arise.
@Test
public void testAddition() {
// Arrange
int a = 5;
int b = 7;
// Act
int result = Calculator.add(a, b);
// Assert
assertEquals(12, result);
}
4. Use Test Doubles
In Android development, you often need to test components that interact with external services or resources, such as databases or APIs. In order to isolate your code from these external dependencies, use test doubles like mocks or stubs. Furthermore, libraries like Mockito can help create these doubles and simulate interactions with external services.
5. UI Testing with Espresso
For the purpose of testing Android app UI components, Google’s Espresso framework is the go-to choice. Basically, Espresso provides a simple and powerful API for interacting with UI elements and validating their behavior. So, write UI tests to ensure that user interactions produce the expected results.
6. Parameterized Tests
JUnit supports parameterized tests, which allow you to run the same test method with different input values. Use parameterized tests when you have multiple inputs and want to ensure consistent behavior across all scenarios.
@ParameterizedTest
@ValueSource(ints = {1, 2, 3, 4, 5})
public void testIsEven(int value) {
assertTrue(value % 2 == 0);
}
7. Continuous Integration (CI) Integration
Integrate JUnit tests into your CI/CD pipeline to automate testing. Jenkins, Travis CI, and other CI tools can be configured to run your tests on every code commit, ensuring that new changes don’t introduce regressions.
8. Code Coverage Analysis
Also, use code coverage analysis tools like JaCoCo to measure the coverage of your tests. Aim for high code coverage to ensure that your tests exercise most of your codebase.
9. Keep Tests Simple and Maintainable
Avoid overly complex test scenarios. Keep your tests simple, focused, and easy to maintain. Complex tests can become a maintenance burden and make it challenging to identify the root cause of failures.
10. Test Across Different Android Versions
Test your app on different Android versions and devices. Android fragmentation can lead to unexpected issues on specific devices or OS versions, so ensure your tests cover a wide range of scenarios.
Conclusion
In conclusion, JUnit is a powerful tool for ensuring the quality of your Android applications. By following these best practices, you can write effective and reliable tests that help you catch and fix issues early in the development process, ultimately leading to a more robust and stable Android app.
Happy testing!
Next: Troubleshooting JUnit Tests
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
100+ MCQs On Java Architecture
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
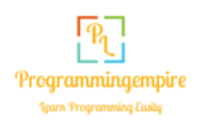