Since JavaScript is one of the popular languages for coding, learners should practice it by writing some code. These beginner-level level JavaScript Practice Exercise questions help them learn the language.
Programmingempire
JavaScript Practice Exercise
- Use JavaScript to display pop up boxes (alert box, an alert box with line breaks, confirm box, prompt box)
- Use JavaScript to call a function with an argument that returns a value.
- Create a web page to demonstrate Java Script events.
- Create a web page to demonstrate Java Script Exception handling.
- Write a JavaScript to accept your name and display it.
- Write a JavaScript code to accept 10 numbers and check how many are +ive, -ive, and zero.
- Write a Program in JavaScript to find the factorial of a number between 1 to 10
Output should be like as given below: –
Factorial of 1 is: 1
Factorial of 2 is: 2
:::::::::::::::::::::::::
::::::::::::::::::::::::::
::::::::::::::::::::::::::
factorial of 10 is :
- Write a Program in JavaScript to find the reverse of a digit.
- Find whether the given number is a strong number or not.
- Display all strong numbers in the given range.
- Write a JavaScript code block, which greets a user with a message Welcome’. When a user leaves this page, the ‘Good-bye’ alert dialog box is displayed
- When user selects a color from the drop-down list, change the background color of a div.
- Demonstrate onblur and onfocus event handlers.
- How to handle mouse events using JavaScript? Demonstrate using a example.
- Write a script that displays the various Math & String Functions.
- Create a student registration form and show the form details. After clicking on submit button following validations must be performed:
- Clear the previous value when a field is selected.
- Display warning message when a field is left blank.
Perform validation on some field against a specified range (onchange event).
- Create a web page that uses JavaScript to change the content of a paragraph.
- How to add a bulleted list in a paragraph each time user clicks on a button.
- After that, add a list with specific ID to a paragraph.
- Write a JavaScript program using a function that adds and removes a bulleted list with the click of buttons.
- Create a web page with input validation using JavaScript.
- Write a JavaScript function that finds both minimum and maximum from an array.
- Create a JavaScript function to sort elements of an array.
- Perform Linear Search and Binary search using JavaScript.
- Demonstrate the use of Arrow functions in JavaScript.
- Find the area of a circle using an anonymous function in JavaScript.
- Change the background color of a paragraph randomly using JavaScript.
Further Reading
Evolution of JavaScript from ES1 to ES2020
Introduction to HTML DOM Methods in JavaScript
Understanding Document Object Model (DOM) in JavaScript
Understanding HTTP Requests and Responses
What is Asynchronous JavaScript?
JavaScript Code for Event Handling
Callback Functions in JavaScript
Show or Hide TextBox when Selection Changed in JavaScript
Changing Style of HTML Elements using getElementsByClassName() Method
Creating Classes in JavaScript
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
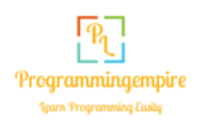