The following example code demonstrates How to Add a Bulleted List to a Paragraph with a Specific ID.
See the previous Example: https://www.programmingempire.com/how-to-add-a-bulleted-list-in-a-paragraph-using-javascript/
The following program also creates a bulleted list in the method addList(). When user clicks on the button, the code adds the list to the paragraph. However, once it is added to the paragraph, it doesn’t add another instance of the list. Because, here, we also add the ID of the list. Before, creating and adding the list, we check whether the list is already there or not. For this purpose, we check whether the list with ID ‘mylist’ is null or not. If it is null, we proceed to create and add the list. Otherwise, we don’t create it.
<!DOCTYPE html>
<html>
<head>
<title>JavaScript createElement</title>
<script>
function addList()
{
var para=document.getElementById('p1');
str1='First Item';
str2='Second Item';
str3='Third Item';
var mylist=document.getElementById('mylist');
if(mylist==null){
var list1=document.createElement('UL');
list1.id='mylist';
var item=document.createElement('LI');
var text=document.createTextNode(str1);
item.appendChild(text);
list1.appendChild(item);
var item=document.createElement('LI');
var text=document.createTextNode(str2);
item.appendChild(text);
list1.appendChild(item);
var item=document.createElement('LI');
var text=document.createTextNode(str3);
item.appendChild(text);
list1.appendChild(item);
para.appendChild(list1);
}
para.innerHTML+="Bulleted List with ID: mylist";
}
</script>
<body>
<p id='p1'></p>
<button onclick="addList()">Add Bulleted List</button>
</body>
</html>
Output
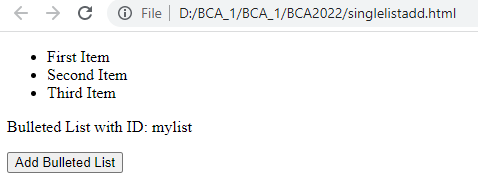
Further Reading
Evolution of JavaScript from ES1 to ES2020
Introduction to HTML DOM Methods in JavaScript
Creating Classes in JavaScript
- AI
- Android
- Angular
- ASP.NET
- Augmented Reality
- AWS
- Bioinformatics
- Biometrics
- Blockchain
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Cyber Security
- Data Science
- Data Structures and Algorithms
- Data Visualization
- Datafication
- Deep Learning
- DevOps
- Digital Forensic
- Digital Trust
- Digital Twins
- Django
- Docker
- Dot Net Framework
- Drones
- Elasticsearch
- ES6
- Extended Reality
- Flutter and Dart
- Full Stack Development
- Git
- Go
- HTML
- Image Processing
- IoT
- IT
- Java
- JavaScript
- Kotlin
- Latex
- Machine Learning
- MEAN Stack
- MERN Stack
- Microservices
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Quantum Computing
- React
- Robotics
- Rust
- Scratch 3.0
- Shell Script
- Smart City
- Software
- Solidity
- SQL
- SQLite
- Tecgnology
- Tkinter
- TypeScript
- VB.NET
- Virtual Reality
- Web Designing
- WebAssembly
- XML
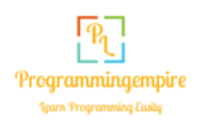