Programmingempire
In this article on Creating Classes in JavaScript, I will explain how to create and use classes in JavaScript. In fact, ES6 introduces classes in JavaScript. Basically, a class encapsulates data and associated methods. The following code shows the syntax of a class in javaScript.
Syntax of a JavaScript Class
class class-name{
constructor(parameters){
.....
}
method-name-1(parameters){
.....
}
method-name-2(parameters){
.....
}
.
.
.
method-name-n(parameters){
.....
}
}
Examples of Creating Classes in JavaScript
the following section provides some examples of Creating Classes in JavaScript. As can be seen in the code below we define a simple class here. Further, the class has only a constructor. In fact, the class has two data string members. So, the constructor initializes them. Also, we can refer to data members using this keyword. In order to create an object of this class, we use the new keyword. The following code shows how to create an object of a class in JavaScript.
Creating Objects
object-name=new class-name(parameters);
For the purpose of creating an object of this class, we use a button. Further, the button handles the onclick event by calling the function f1(). So, we are creating an object of the class within this function.
Example 1 – Creating a Simple Class
<!DOCTYPE html>
<html>
<head>
<title>JavaScript Class Example</title>
<style>
.btn{
background: #dd3388;
color: #ff33ff;
border: 1px #dd3388 solid;
border-radius: 5px;
font-size: 25px;
font-weight: bolder;
margin: 20px;
padding: 25px;
}
</style>
<script>
class JSClass
{
constructor(name, value)
{
this.name=name;
this.value=value;
alert("Class Name: "+name+"\nValue: "+value);
}
}
function f1()
{
ob=new JSClass("JSClass", "A Simple Class");
}
</script>
</head>
<body>
<button class="btn" onclick="f1()">Click Me!</button>
</body>
</html>
Output

More Examples on Creating Classes in JavaScript
The following example shows how to create methods in a JavaScript class. Evidently, a method in a JavaScript class doesn’t use the function keyword. Hence, we define a method in a JavaScript class by just its name and associated parameters. As can be seen, the class has two methods greet(), and welcome().
Example 2 – Defining Methods
<!DOCTYPE html>
<html>
<head>
<title>JavaScript Class Example</title>
<style>
.btn{
background: #FCF83C;
color: #F93B12;
border: 1px #FCF83C solid;
border-radius: 20px;
font-size: 25px;
font-weight: bolder;
margin: 20px;
padding: 15px;
}
.mydiv{
display: inline-block;
}
</style>
<script>
class MyClass
{
constructor(str)
{
this.str=str;
}
greet()
{
alert("Tutorial of JavaScript Classes at programmingempire.com");
}
welcome()
{
alert("Welcome "+ this.str);
}
}
let p=prompt("Enter Your Name: ");
let obj=new MyClass(p);
obj.greet();
obj.welcome();
</script>
</head>
<body>
</body>
</html>
Output
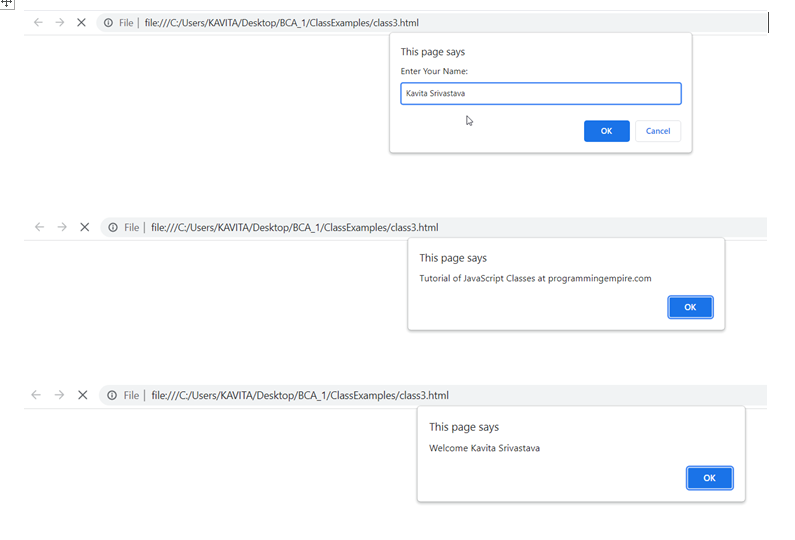
Similarly, the next example shows a class with a constructor and several methods.
Example 3 – Creating Classes in JavaScript cContaining Constructor and Methods
The following example demonstrate a class with name “MathClass”. Also, see the usage of global variables. So, the ob is a global variable that we use to create an object of this class. Since we use ob in different functions, we need a global variable for creating object.
<!DOCTYPE html>
<html>
<head>
<title>JavaScript Class Example</title>
<style>
.btn{
background: #FCF83C;
color: #F93B12;
border: 1px #FCF83C solid;
border-radius: 20px;
font-size: 25px;
font-weight: bolder;
margin: 20px;
padding: 15px;
}
.mydiv{
display: inline-block;
}
</style>
<script>
var x;
var y;
var ob;
class MathClass
{
constructor(a, b)
{
this.a=a;
this.b=b;
}
findsum()
{
alert("Sum = "+(this.a+this.b));
}
finddifference()
{
alert("Sum = "+(this.a-this.b));
}
findproduct()
{
alert("Sum = "+(this.a*this.b));
}
finddivision()
{
alert("Sum = "+(this.a/this.b));
}
}
function getInput()
{
x=prompt("Enter First Number: ");
y=prompt("Enter Second Number: ");
x=Number(x);
y=Number(y);
ob=new MathClass(x, y);
}
getInput();
function f1()
{
ob=new MathClass(x, y);
ob.findsum();
}
function f2()
{
ob=new MathClass(x, y);
ob.finddifference();
}
function f3()
{
ob=new MathClass(x, y);
ob.findproduct();
}
function f4()
{
ob=new MathClass(x, y);
ob.finddivision();
}
</script>
</head>
<body>
<div class="mydiv">
<button class="btn" onclick="f1()">Find Sum</button>
</div>
<div class="mydiv">
<button class="btn" onclick="f2()">Find Difference</button>
</div>
<div class="mydiv">
<button class="btn" onclick="f3()">Find Product</button>
</div>
<div class="mydiv">
<button class="btn" onclick="f4()">Find Division</button>
</div>
</body>
</html>
Output

Further Reading
Evolution of JavaScript from ES1 to ES2020
Introduction to HTML DOM Methods in JavaScript
Understanding Document Object Model (DOM) in JavaScript
JavaScript Code for Event Handling
Understanding HTTP Requests and Responses
What is Asynchronous JavaScript?
Callback Functions in JavaScript
Show or Hide TextBox when Selection Changed in JavaScript
Changing Style of HTML Elements using getElementsByClassName() Method
Creating Classes in JavaScript
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
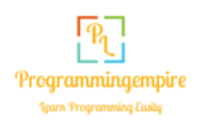