Programmingempire
In general Asynchronous JavaScript lets the code execute immediately without blocking. For the purpose of understanding this concept let us assume that we have two statements in sequential order in a code. While the first statement being executed, the second one will wait for it to finish. It is known as synchronous execution.
On the other hand, if the second statement starts executing while the first one is still in progress, it is called asynchronous execution. For instance, suppose we have a database operation that writes several records in database doesn’t effect the future operations. In such a case it is possible to initiate the later operations without waiting for a time-consuming operation. Hence, the asynchronous code is the one that starts now and can finish later. So, the asynchronous functions in JavaScript make the code non-blocking.
Example of Asynchronous JavaScript Code
To illustrate the above concept let us take an example. The folowing code shows four function calls that will execute in the synchronous manner. As can be seen in the output all the functions execute sequentially.
<script>
document.write("These are all");
document.write("<br/>Synchronous statements!");
document.write("<br/>They will be called");
document.write("<br/>One after another");
</script>
Output

Further, we modify the code as shown below. Basically, the setTimeout() function takes a callback function as a parameter. Further, it takes the time in milliseconds as another parameter. Therefore, the corresponding function call for the callback function takes place after the given time is elapsed.
<script>
document.write("These are all");
document.write("<br/>Synchronous statements!");
setTimeout(()=>{
document.write("<br>It is a non-blockinh call");
document.write("<br>that will finish after 5 seconds!");
}, 5000);
document.write("<br/>They will be called");
document.write("<br/>One after another");
</script>
The following output will be displayed after 5 seconds of the output shown earlier.

Async/Await
Basically, Async and Await are two keywords in JavaScript that are introduced recently. While the Async operates asynchronously via the event loop. Also, it returns a value. Further, it ensures that a Promise is returned. Another function is Await. As can be seen we can use the Await function to make the function call wait until the Promise is returned. Hence, it is an async blocking wait. Furthermore, Promises in JavaScript are nothing but the processes that we can chain using callback functions.
Further Reading
Evolution of JavaScript from ES1 to ES2020
Introduction to HTML DOM Methods in JavaScript
Understanding Document Object Model (DOM) in JavaScript
Understanding HTTP Requests and Responses
What is Asynchronous JavaScript?
JavaScript Code for Event Handling
Callback Functions in JavaScript
Show or Hide TextBox when Selection Changed in JavaScript
Changing Style of HTML Elements using getElementsByClassName() Method
Creating Classes in JavaScript
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
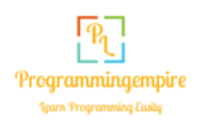