In this article, I will explain Creating User-Defined Functions in javaScript. Since many times we need to use the same program statements at several places in a program. Therefore, we create functions that perform a specific task. These functions are called user-defined functions. Once, we have a function for performing a specific task, we can use it anywhere in the program.
In JavaScript, we create a function using the function keyword. Also, the functions may or may not take arguments. Furthermore, a function may also return a value. In order to pass the argument, we use the values or variables in the parentheses that follow the function name in a function call. These are the actual arguments. Similarly, in the function definition, we use certain variable names in the parentheses that hold the value of actual arguments. These variables are the formal arguments. Similarly, we use the return keyword to return a value.
Programmingempire
An Example for Creating User-Defined Functions in javaScript
The following HTML code contains JavaScript functions. As can be seen, the first function doesn’t take any argument. Also, it has no return value. In order to call the function, we just use the name of the function followed by parentheses. Similarly, we define another function that returns a value. After that, we define a function that takes two arguments. Since JavaScript is not a strictly typed language, we don’t specify the data types. Lastly, we have a function that takes parameters as well as returns a value.
<html>
<head>
<title>User-Defined Functions</title>
<script>
function func1()
{
document.write("This is produced by a no-argument, no-return value function!");
}
function func2()
{
document.write("<br/>This is produced by a no-argument function that returns a value!");
str=prompt("Enter a String: ", "start typing here...");
return str;
}
function func3(a, b)
{
document.write("<br/>This is produced by a function that takes two parameters and doesn't return a value!");
document.write(a*a+b*b);
}
function func4(a, b)
{
document.write("<br/>This is produced by a function that takes two parameters and returns a value!");
return a*a-b*b;
}
func1();
document.write(func2());
func3(7, 9);
document.write(func4(7, 9));
</script>
</head>
<body>
<div style="text-align:center;">
<h1>JavaScript Example to Demonstrate User-Defined functions!</h1>
</div>
</body>
</html>
Output

Further Reading
Evolution of JavaScript from ES1 to ES2020
Introduction to HTML DOM Methods in JavaScript
Understanding Document Object Model (DOM) in JavaScript
What is Asynchronous JavaScript?
JavaScript Code for Event Handling
Callback Functions in JavaScript
Understanding HTTP Requests and Responses
Show or Hide TextBox when Selection Changed in JavaScript
Changing Style of HTML Elements using getElementsByClassName() Method
Creating Classes in JavaScript
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
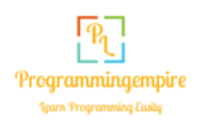