The following example demonstrates how the Popup Boxes in JavaScript work. Basically, JavaScript offers three popup boxes. In order to display a warning message, we use the alert popup box. It displays a warning message along with an OK button. In order to proceed further, the user requires to press the OK button.
Similarly, we have another popup box called the confirm box. Basically, we use the confirm popup box when we need to grant some permission to the user. For instance, suppose, the user is downloading a certain file. Then, a confirm popup box can display a confirmation message. Apart from the confirmation message, the confirm popup box also displays the OK and Cancel buttons. So, the operation is performed only when the user presses the OK button. Otherwise, it cancels that operation.
Likewise, we have a prompt popup box. Unlike the other two popup boxes, the prompt box accepts input from the user. Hence, we use the prompt box in order to take input values. Further, we can use the values elsewhere in the program.
The following HTML page contains JavaScript code that contains the above three popup boxes. Also, the input value that we retrieve from the user with the prompt box is displayed by an alert box.
Programmingempire
Example to Demonstrate Popup Boxes in JavaScript
<html>
<head>
<title>Popup Boxes in JavaScript</title>
<script>
alert("This is an example of alert box!");
confirm("It is a Confirm popup box!");
var myvariable=prompt("This is a prompt box!", "Enter someting....");
alert("You have entered: "+myvariable);
</script>
</head>
<body>
<div style="text-align:center;">
<h1>JavaScript Example to Demonstrate Popup Boxes!</h1>
</div>
</body>
</html>
Output

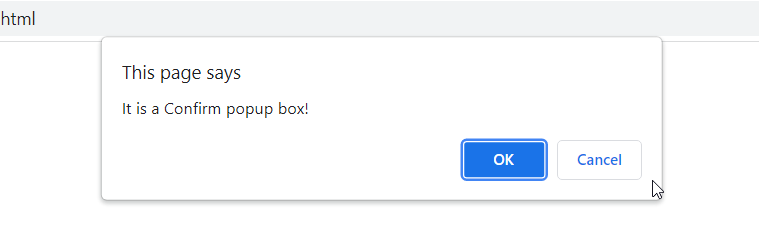

Further Reading
Evolution of JavaScript from ES1 to ES2020
Introduction to HTML DOM Methods in JavaScript
Understanding HTTP Requests and Responses
What is Asynchronous JavaScript?
JavaScript Code for Event Handling
Callback Functions in JavaScript
Understanding Document Object Model (DOM) in JavaScript
Show or Hide TextBox when Selection Changed in JavaScript
Changing Style of HTML Elements using getElementsByClassName() Method
Creating Classes in JavaScript
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
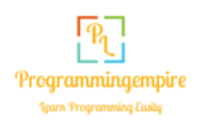