Programmingempire
To summarize the validation errors on a web page we use Validation Summary Control in ASP.NET. However, for a basic understanding of server-side validation controls, you can read this article.
Important Points Regarding the validation Summary Control
- In fact, this control doesn’t perform any validation itself but only collects error information from other validation controls and displays it in a specified format.
- Instead of using it as a stand-alone control, the ValidationSummary control is used along with other validation controls.
- Furthermore, This control displays the consolidated error messages on a web page.
- Since you get complete error information in one place, there is no need to look at error messages produced by individual validation controls.
- For the purpose of displaying error information, the ValidationSummary control uses the ErrorMessage property of individual validation controls.
Example of Validation Summary Control
As an illustration, we use the ValidationSummary control along with three more validation controls that we have used in the previous example. Although, it is one of the simplest and easy to use control in ASP.NET, evidently its use on a web page makes the page attractive and user-friendly.
Specifically, the example uses two more properties along with the styling properties. Firstly, the HeaderText property specifies the text that is displayed on the top. Similarly, the DisplayMode property specifies the format in which the summary should be displayed. For example, the value “BulletedList” shows error messages in a bulleted list. While other possible values of the DisplayMode property are List and SingleParagraph.
Another key point is that you can also show the error summary using a Message Box. For this purpose, it has a property called ShowMessageBox that you can set to true.
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="WebForm1.aspx.cs" Inherits="ValidationControlExamples.WebForm1" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
Enter Some Text: *<asp:TextBox ID="TextBox1" runat="server"></asp:TextBox><br />
<asp:RequiredFieldValidator ID="r1" runat="server"
ErrorMessage="The Field Can't be Left Blank"
ControlToValidate="TextBox1"
BackColor="LightYellow"
Display="Static"
Text="This Field is Mandatory!"
></asp:RequiredFieldValidator>
<hr />
Comparing the Content of Two Fields<br />
Enter Text: <asp:TextBox ID="TextBox2" runat="server"></asp:TextBox><br />
Enter Text Again: <asp:TextBox ID="TextBox3" runat="server"></asp:TextBox><br />
<asp:CompareValidator ID="c1" runat="server"
ErrorMessage="Two Values don't match"
BackColor="LightBlue"
Display="Static"
Operator="Equal"
Type="String"
Text="Mismatch!"
ControlToValidate="TextBox2"
ControlToCompare="TextBox3"
></asp:CompareValidator>
<hr />
Validating Range
Enter a number [10-100]: <asp:TextBox ID="TextBox4" runat="server"></asp:TextBox><br />
<asp:RangeValidator ID="r2" runat="server"
ErrorMessage="Invalid Range!"
Text="Value Not in Given Range!"
BackColor="LightCoral"
Display="Static"
MaximumValue="100"
MinimumValue="10"
Type="Integer"
ControlToValidate="TextBox4"
></asp:RangeValidator>
<asp:Button
id="Button2"
text="Validate"
runat="server" />
<asp:ValidationSummary
id="ValidationSummary1"
DisplayMode="BulletList"
runat="server"
HeaderText="Errors on the page!"
Font-Names="verdana"
Font-Size="12"/>
<hr />
</div>
</form>
</body>
</html>
Output

Summary
To sum up, the ValidationSummary controls is one of the important control available in ASP.NET. Also, it increases the readability of the web page considerably. Unlike other validation controls, we don’t use it stand-alone but along with other validation controls. Hence, it consolidates error information from other controls and displays it in a specific part of the web page.
Further Reading
Parameter and ParameterCollection in ADO.NET
Database Manipulation Using DataGrid
Example of Button and Link Button Control in ASP.NET
Example of Chart Control in ASP.NET
Creating a DataTable from a DataReader in ASP.NET
Deleting a Record using DataGrid Control in ASP.NET
Edit a Record Using DataGrid Control in ASP.NET
Insert a Record Using ItemCommand Event in DataGrid
CRUD Operations with DataGrid in ASP.NET
Creating Columns in a DataGrid Control
XML Documents and DataSet in ASP.NET
ASP.NET Core Features and Advantages
Display Images Using DataList Control
Adding Images Using Image Control
Creating a Group of Radio Buttons Using RadioButtonList Control
Example of Button Control in ASP.NET
ItemDataBound Event in DataList
More Features of DataList in ASP.NET
A Simple Example of Using a DataList Control in ASP.NET
Properties and Methods of DataList Control in ASP.NET
Exploring DataList Control in ASP.NET
Custom Validator Control in ASP.NET
Validation Summary Control in ASP.NET
Validation Controls Examples – RequiredFieldValidator, CompareValidator, and RangeValidator
An Example of Data Binding with RadioButtonList Control
Binding Data to Web Control in ADO.NET
Examples of AdRotator Control in ASP.NET
Examples of Validation Controls in ASP.NET
Overview of MVC architecture in ASP.NET
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
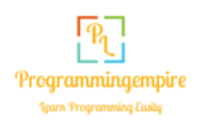