Programmingempire
In this post on Adding Images Using Image Control, I will demonstrate the use of Image Control for creating a Web Page in ASP.NET.
Basically, the Image Control available in ASP.NET allows us to display an image on the web page. Hence, it displays the image as the data. Therefore, we can display images within any container element of HTML such as DIV.
Properties of Image Control
Although Image control has several properties, here some of the important properties are discussed. Significantly, the ImageUrl property that we use to set the path of the image that we wish to display. Generally, we use the ImageAlign property to align the image along with the text.
Likewise, other important properties of Image control are Width, Height, and AlternateText. Also, it has several other properties that we can use to manipulate its appearance like BackColor, BorderColor, BorderStyle, and BorderWidth. However, we can do the same with the help of CSS also. For this purpose, we can use the Attributes property to associate a CSS class with the Image control.
Example of Adding Images Using Image Control
The following example shows how to use the Image control. In order to display images, first we create an ASP.NET Web application and add a Web Form using Visual Studio. After that, we need to add the images in our project that we want to display.
Hence, Select Add Existing Item option from the Project menu. Further, select the images and press OK. Now that, we have images available with us in the project folder. Therefore we can use them to specify the ImageUrl property of the Image control.
WebForm1.aspx
The following code shows six Image controls that we use within a DIV element. As can be seen, we specify only the ImageUrl property in the ASPX file.
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="WebForm1.aspx.cs" Inherits="ImageControlExample.WebForm1" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<style>
.c1
{
background-color: gold;
margin:100px;
padding:50px;
align-items: center;
min-height:400px;
text-align: center;
}
.c2{
margin: 20px;
border: 5px DarkGreen solid;
padding:10px;
}
</style>
</head>
<body>
<form id="form1" runat="server">
<div id="d1" runat="server">
<asp:Image ID="Image1" runat="server" ImageUrl="~/1.jpg" />
<asp:Image ID="Image2" runat="server" ImageUrl="~/2.jpg" />
<asp:Image ID="Image3" runat="server" ImageUrl="~/3.jpg" />
<asp:Image ID="Image4" runat="server" ImageUrl="~/4.jpg" />
<asp:Image ID="Image5" runat="server" ImageUrl="~/5.jpg" />
<asp:Image ID="Image6" runat="server" ImageUrl="~/6.jpg" />
</div>
</form>
</body>
</html>
WebForm1.aspx.cs
The following code-behind file shows how we set other properties of the Image control dynamically. In this case, we set other properties of Image control such as Width, Height, and AlternateText dynamically.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
namespace ImageControlExample
{
public partial class WebForm1 : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
d1.Attributes.Add("class", "c1");
SetDimensions();
SetLayout();
SetProperties();
}
public void SetLayout()
{
Image1.Attributes.Add("class", "c2");
Image2.Attributes.Add("class", "c2");
Image3.Attributes.Add("class", "c2");
Image4.Attributes.Add("class", "c2");
Image5.Attributes.Add("class", "c2");
Image6.Attributes.Add("class", "c2");
}
public void SetDimensions()
{
Image1.Width = 300;
Image1.Height = 280;
Image2.Width = 300;
Image2.Height = 280;
Image3.Width = 300;
Image3.Height = 280;
Image4.Width = 300;
Image4.Height = 280;
Image5.Width = 300;
Image5.Height = 280;
Image6.Width = 300;
Image6.Height = 280;
}
public void SetProperties()
{
Image1.AlternateText = "Tree1";
Image2.AlternateText = "Tree2";
Image3.AlternateText = "Tree3;
Image4.AlternateText = "Tree4";
Image5.AlternateText = "Tree5";
Image6.AlternateText = "Tree6";
}
}
}
Output
The following image shows the output of execution.

To sum up, we can use Image control to display images on a web page. Although, we can use it independently of any other control. Still, it can be used as part of other controls such as in DataList control. In fact, the next example demonstrates how Image control can be used as a part of DataList control.
Further Reading
Parameter and ParameterCollection in ADO.NET
Database Manipulation Using DataGrid
Example of Button and Link Button Control in ASP.NET
Example of Chart Control in ASP.NET
Creating a DataTable from a DataReader in ASP.NET
Deleting a Record using DataGrid Control in ASP.NET
Edit a Record Using DataGrid Control in ASP.NET
Insert a Record Using ItemCommand Event in DataGrid
CRUD Operations with DataGrid in ASP.NET
Creating Columns in a DataGrid Control
XML Documents and DataSet in ASP.NET
ASP.NET Core Features and Advantages
Display Images Using DataList Control
Adding Images Using Image Control
Creating a Group of Radio Buttons Using RadioButtonList Control
Example of Button Control in ASP.NET
ItemDataBound Event in DataList
More Features of DataList in ASP.NET
A Simple Example of Using a DataList Control in ASP.NET
Properties and Methods of DataList Control in ASP.NET
Exploring DataList Control in ASP.NET
Custom Validator Control in ASP.NET
Validation Summary Control in ASP.NET
Validation Controls Examples – RequiredFieldValidator, CompareValidator, and RangeValidator
An Example of Data Binding with RadioButtonList Control
Binding Data to Web Control in ADO.NET
Examples of AdRotator Control in ASP.NET
Examples of Validation Controls in ASP.NET
Overview of MVC architecture in ASP.NET
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
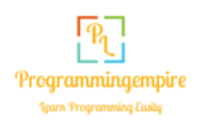