The following program demonstrates How to Create and Remove a Bulleted List Using JavaScript.
See More examples on adding HTML elements dynamically:
https://www.programmingempire.com/how-to-add-a-bulleted-list-in-a-paragraph-using-javascript/
https://www.programmingempire.com/how-to-add-a-bulleted-list-to-a-paragraph-with-a-specific-id/
The following program contains functions for both adding the list as well as removing the list. So, here we use the ID ‘mylist’ to add and remove the list. In order to do so, we use the DOM methods. While the createElement() method creates an HTML element. Also, the appendChild() method adds a child element t the specified element. Similarly, removeChild() method removes an element with specific id. Also, note the use of getElementById() method.
Basically, we use the getElementById() method to obtain a reference to an element.
<!DOCTYPE html>
<html>
<head>
<title>JavaScript createElement</title>
<script>
function addList()
{
var para=document.getElementById('p1');
para.innerHTML="";
str1='First Item';
str2='Second Item';
str3='Third Item';
var mylist=document.getElementById('mylist');
if(mylist==null){
var list1=document.createElement('UL');
list1.id='mylist';
var item=document.createElement('LI');
var text=document.createTextNode(str1);
item.appendChild(text);
list1.appendChild(item);
var item=document.createElement('LI');
var text=document.createTextNode(str2);
item.appendChild(text);
list1.appendChild(item);
var item=document.createElement('LI');
var text=document.createTextNode(str3);
item.appendChild(text);
list1.appendChild(item);
para.appendChild(list1);
}
para.innerHTML+="Bulleted List with ID: mylist";
}
function removeList()
{
var para=document.getElementById('p1');
var list=document.getElementById('mylist');
if(list!=null)
{
para.removeChild(list);
}
para.innerHTML="Bulleted List Removed";
}
</script>
<body>
<p id='p1'></p>
<button onclick="addList()">Add Bulleted List</button>
<button onclick="removeList()">Remove Bulleted List</button>
</body>
</html>
Output
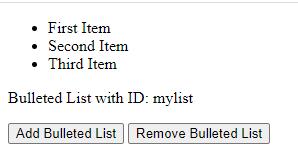

Further Reading
Evolution of JavaScript from ES1 to ES2020
Introduction to HTML DOM Methods in JavaScript
Creating Classes in JavaScript
- AI
- Android
- Angular
- ASP.NET
- Augmented Reality
- AWS
- Bioinformatics
- Biometrics
- Blockchain
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Cyber Security
- Data Science
- Data Structures and Algorithms
- Data Visualization
- Datafication
- Deep Learning
- DevOps
- Digital Forensic
- Digital Trust
- Digital Twins
- Django
- Docker
- Dot Net Framework
- Drones
- Elasticsearch
- ES6
- Extended Reality
- Flutter and Dart
- Full Stack Development
- Git
- Go
- HTML
- Image Processing
- IoT
- IT
- Java
- JavaScript
- Kotlin
- Latex
- Machine Learning
- MEAN Stack
- MERN Stack
- Microservices
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Quantum Computing
- React
- Robotics
- Rust
- Scratch 3.0
- Shell Script
- Smart City
- Software
- Solidity
- SQL
- SQLite
- Tecgnology
- Tkinter
- TypeScript
- VB.NET
- Virtual Reality
- Web Designing
- WebAssembly
- XML
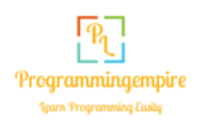