The following code shows an Example of Arrow Function in JavaScript.
Basically, we use arrow functions when we need to write a concise function. It represents a shorter syntax of the function. The following code example shows an arrow function to find the power of a number.
<html>
<head>
<title>Arrow Functions in JavaScript</title>
<script>
let powerFunction = (x, y) => {
p=1;
for(i=1;i<=y;i++)
{
p=p*x;
}
return p;
};
function findPower()
{
a=Number(prompt('Enter the value of x: '));
b=Number(prompt('Enter the value of y: '));
alert(a+' raise to the power '+b+' is '+powerFunction(a, b));
}
</script>
<style>
div{
margin: 100px;
padding: 50px;
border: 2px black solid;
text-align: center;
}
</style>
</head>
<body>
<div>
<button onclick="findPower()">Find Power</button>
</div>
</body>
</html>
Output

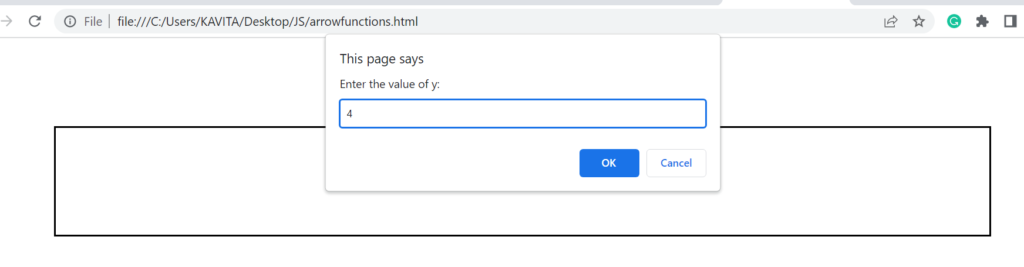
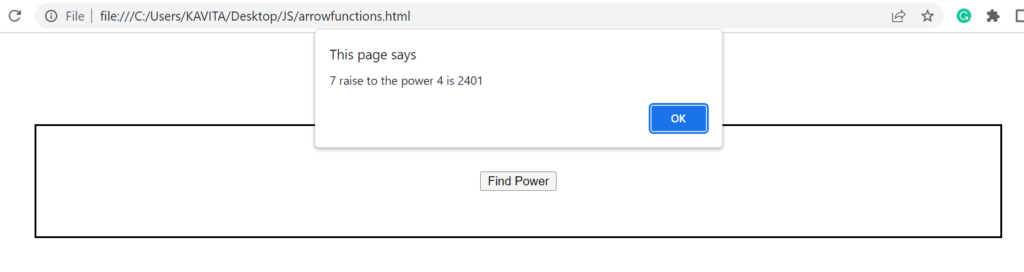
Further Reading
Evolution of JavaScript from ES1 to ES2020
Introduction to HTML DOM Methods in JavaScript
Understanding Document Object Model (DOM) in JavaScript
What is Asynchronous JavaScript?
Understanding HTTP Requests and Responses
- Angular
- ASP.NET
- Bootstrap
- C
- C#
- C++
- CSS
- Deep Learning
- Django
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- MEAN Stack
- MERN Stack
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Scratch 3.0
- SQL
- TypeScript
- VB.NET
- Web Designing
- XML
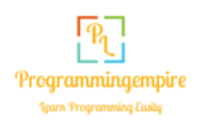