The following example code demonstrates How to Find Minimum and Maximum from an Array in JavaScript.
At first, we create an array of ten elements. Also, note that we have three buttons on the web page. While the first button, when clicked, takes ten numbers from the user as input. Similarly, the second button when clicked, displays the elements of the array. In order to find minimum and maximum from the array elements, first we set minimum and maximum as the first element of the array. After that we start a for loop that iterates over all elements starting from the second one and find the minimum and maximum. When user clicks on the third button, the minimum and maximum are displayed in another paragraph.
<html>
<head>
<title>Sorting in JavaScript</title>
<script>
arr=Array(10);
function findMinimumMaximum()
{
min_element=arr[0];
max_element=arr[0];
n=10;
for(i=1;i<n;i++)
{
if(arr[i]<min_element) min_element=arr[i];
if(arr[i]>max_element) max_element=arr[i];
}
str="Minimum Element: "+min_element;
str+="<br>Maximum Element: "+max_element;
var x=document.getElementById('d2');
x.innerHTML+=str;
}
function inputElements()
{
for(i=0;i<10;i++)
{
arr[i]=Number(prompt('Enter Element '+(i+1)));
}
}
function displayElements()
{
var str="";
for(i=0;i<10;i++)
{
str+=arr[i]+" ";
}
var x=document.getElementById('d1');
x.innerHTML+=str;
}
</script>
<style>
div{
margin: 100px;
padding: 50px;
border: 2px black solid;
text-align: center;
}
</style>
</head>
<body>
<div>
<button onclick="inputElements()">Input Elements</button>
<button onclick="displayElements()">Display Elements</button>
<button onclick="findMinimumMaximum()">Find Minimum and Maximum</button>
<p id="d1">Array Elements: </p>
<p id="d2"></p>
</div>
</body>
</html>
Output
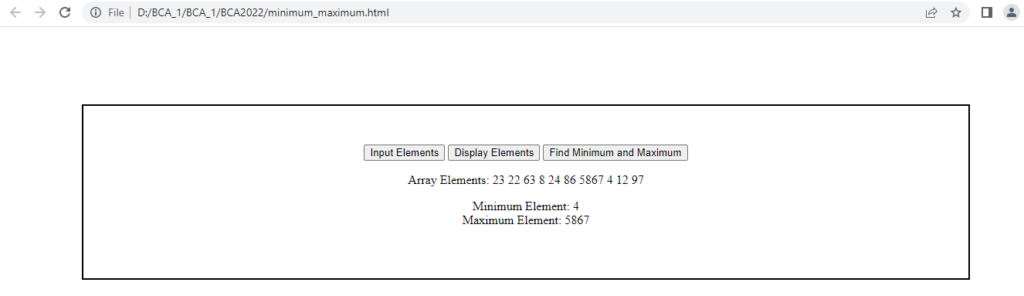
Further Reading
Evolution of JavaScript from ES1 to ES2020
Introduction to HTML DOM Methods in JavaScript
Understanding Document Object Model (DOM) in JavaScript
What is Asynchronous JavaScript?
Understanding HTTP Requests and Responses
- Angular
- ASP.NET
- Bootstrap
- C
- C#
- C++
- CSS
- Deep Learning
- Django
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- MEAN Stack
- MERN Stack
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Scratch 3.0
- SQL
- TypeScript
- VB.NET
- Web Designing
- XML
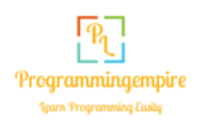