Programmingempire
In this article, I will explain how to use An array of Objects and Object Initializer in C#. To begin with, let us first understand what is an array.
Basically, an array is a collection of elements, all of which have the same data type. Further, in a programming language such as C#, we can have both the built-in types as well as user-defined types. Therefore, C# has built-in types like int, float, double, char, boolean, decimal, and so on. Likewise, C# also has user-defined types such as classes and struct.
Furthermore, C# categorizes data types as Value Types and Reference Types. While the C# compiler allocates value types statically, the runtime system allocates reference types dynamically. As a matter of fact, the compiler allocates all the built-in types along with the struct type statically. Hence, these types are Value Types. In contrast, types like array, classes, interface, and delegates are dynamic. Because CLR allocates memory dynamically to these types. ally allocated. Therefore, these types are reference types.
Also, in C# all types have a base class known as Object, regardless of whether it is a value type or a reference type.
Object Initializer in C#
A class in C# encapsulates the attributes and methods. Further, in C# we call the attributes as fields. Also, a class also has a member known as a constructor that we use to initialize the fields of the class. However, in case the class doesn’t define a constructor, we can use the object initializer to assign initial values to the fields of the class. The following code shows the syntax of the object initializer.
new ClassName{field1=value1, field2=value2, ......, fieldn=valuen};
Example of Using An array of Objects and Object Initializer
The following example demonstrates how to use An array of Objects and Object Initializer. As can be seen, in this example, we have a class called Skills that represents the skill requirement of an organization. Also, the array of objects uses the object initializer to initialize the individual object. Finally, the array is displayed using the foreach loop.
using System;
namespace ObjectArray
{
class Skills
{
public String category;
public String skillname;
public String expertise_level;
public override string ToString()
{
String str = @$"Skill Category: {category}
Skill: {skillname}
Expertise Level: {expertise_level}";
return str+"\n";
}
}
class Program
{
static void Main(string[] args)
{
//Creating Object Array using Object Initializer
Skills[] skarr = { new Skills {category="Technical", skillname="PHP", expertise_level="High"},
new Skills {category="Non-Technical", skillname="Technical Writing", expertise_level="Medium"},
new Skills {category="Non-Technical", skillname="Time-Management", expertise_level="Low"},
new Skills {category="Technical", skillname="NodeJs", expertise_level="Medium"},
new Skills {category="Non-Technical", skillname="Collaboration", expertise_level="Low"},
new Skills {category="Non-Technical", skillname="Leadership", expertise_level="Medium"},
new Skills {category="Technical", skillname="ASP.NET", expertise_level="High"},
new Skills {category="Technical", skillname="Java", expertise_level="Medium"},
new Skills {category="Technical", skillname="Angular", expertise_level="High"},
new Skills {category="Technical", skillname="JavaScript", expertise_level="High"},
};
Console.WriteLine("Skills Required in Our Organization....");
Console.WriteLine("---------------------------------------\n");
foreach (Skills s in skarr)
{
Console.WriteLine(s);
}
}
}
}
Output
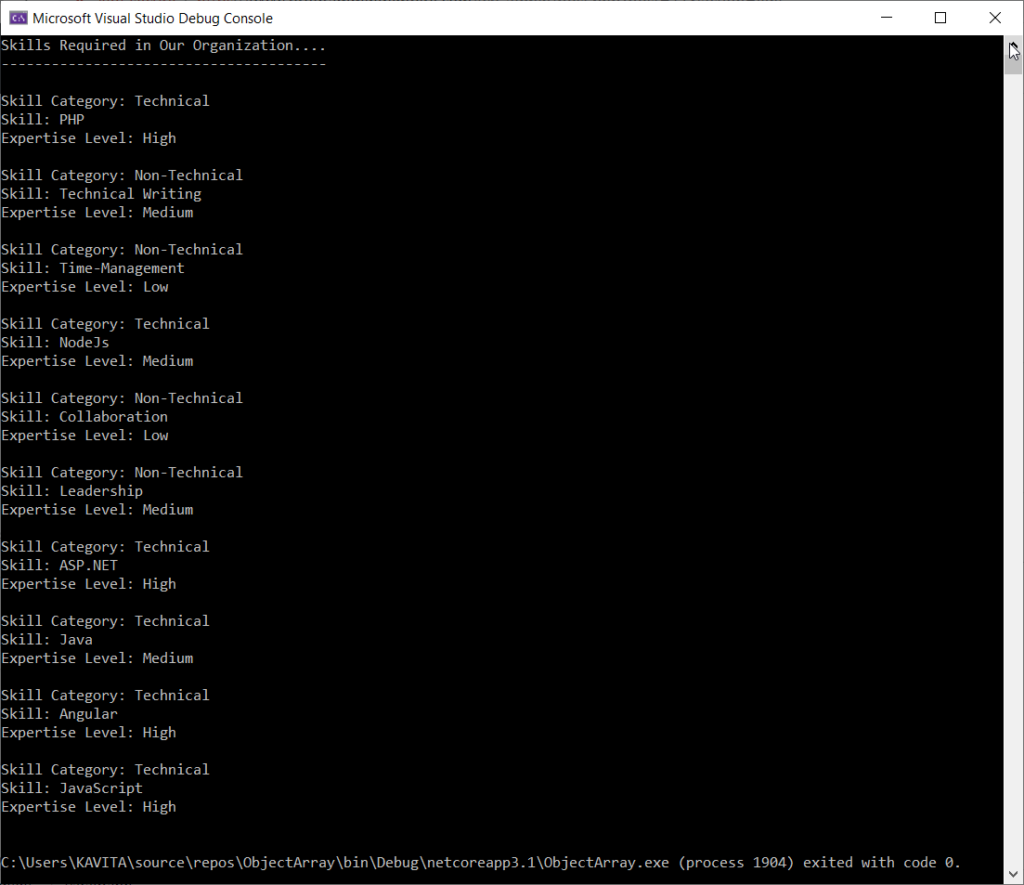
Further Reading
How to Create Instance Variables and Class Variables in Python
Comparing Rows of Two Tables with ADO.NET
Example of Label and Textbox Control in ASP.NET
One Dimensional and Two Dimensuonal Indexers in C#
Private and Static Constructors in C#
Programs to Find Armstrong Numbers in C#
One Dimensional and Two Dimensional Indexers in C#
Generic IList Interface and its Implementation in C#
Creating Navigation Window Application Using WPF in C#
Find Intersection Using Arrays
An array of Objects and Object Initializer
Performing Set Operations in LINQ
Data Binding Using BulletedList Control
Understanding the Quantifiers in LINQ
Deferred Query Execution and Immediate Query Execution in LINQ
Examples of Query Operations using LINQ in C#
An array of Objects and Object Initializer
Language-Integrated Query (LINQ) in C#
Examples of Connected and Disconnected Approach in ADO.NET
IEnumerable and IEnumerator Interfaces
KeyValuePair and its Applications
Learning All Class Members in C#
Examples of Extension Methods in C#
How to Setup a Connection with SQL Server Database in Visual Studio
Understanding the Concept of Nested Classes in C#
A Beginner’s Tutorial on WPF in C#
Explaining C# Records with Examples
Everything about Tuples in C# and When to Use?
Linear Search and Binary search in C#
Examples of Static Constructors in C#
When should We Use Private Constructors?
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
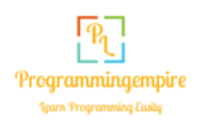