Programmingempire
In this post, I will answer the question When should We Use Private Constructors? For the basic information on private constructors, you can read this post on Private and Static Constructors in C#.
As you already know the basic purpose of defining a constructor in a class is to create the instance of that class. Further, as we generally instantiate a class, outside of its own definition, we declare constructors as public.
But you must remember that a class is a concept in an object-oriented paradigm and the objects are the real-world entities that have ceratin features and a well-defined behavior. Still, there are many applications in which we don’t deal with real-world objects. However, object-oriented languages like C# make it mandatory to write the code in a class only.
To illustrate further, suppose we want to create a class which provides several mathemetical functions. These functions are mostly static and called with the name of class and dot operator. This class of mathematical operationss doesn’t represent any real-world entity. In such case, we should restrict user to create an object of this class. Therefore, we should make the constructor private in such case.
Consider another example of a class that contains functions for computing factorial of a number.
using System;
namespace PrivateConstructorDemo
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine(FactorialFunctions.Factorial(6));
Console.WriteLine(FactorialFunctions.RecursiveFactorial(9));
}
}
class FactorialFunctions
{
public static int Factorial(int v)
{
int fact = 1;
if (v == 0) return 1;
for (int i = 1; i <= v; i++)
{
fact = fact * i;
}
return fact;
}
public static int RecursiveFactorial(int v)
{
if (v == 0)
return 1;
else
return v * RecursiveFactorial(v - 1);
}
}
}
The class FactorialFunctions contains only two static methods. Now, let us create object of this class:
static void Main(string[] args)
{
FactorialFunctions ob = new FactorialFunctions();
Console.WriteLine(FactorialFunctions.Factorial(6));
Console.WriteLine(FactorialFunctions.RecursiveFactorial(9));
}
The above code will compile without error since the compiler provides a default constructor to a class if the programmer doesn’t define one. However, it doesn’t make sense to instantiate this class, since it doesn’t have any non-static member. Therefore, by making the constructor private, we can prevent creating an instance of this class, as shown below.
using System;
namespace PrivateConstructorDemo
{
class Program
{
static void Main(string[] args)
{
// FactorialFunctions ob = new FactorialFunctions();
Console.WriteLine(FactorialFunctions.Factorial(6));
Console.WriteLine(FactorialFunctions.RecursiveFactorial(9));
}
}
class FactorialFunctions
{
FactorialFunctions() { }
public static int Factorial(int v)
{
int fact = 1;
if (v == 0) return 1;
for (int i = 1; i <= v; i++)
{
fact = fact * i;
}
return fact;
}
public static int RecursiveFactorial(int v)
{
if (v == 0)
return 1;
else
return v * RecursiveFactorial(v - 1);
}
}
Now, let us summarize important points regarding the usage of private constructors.
- We can use private constructor in a class if it has no instance member.
- Use of private constructor prevents a class from being inherited.
- Private constructors are used to create a singleton class.
- If a class contains static factory methods to create instance of that class, then it should have private constructors.
n this post, I will answer the question When should We Use Private Constructors? For the basic information on private constructors, you can read this post on Private and Static Constructors in C#.
As you already know the basic purpose of defining a constructor in a class is to create the instance of that class. Further, as we generally instantiate a class, outside of its own definition, we declare constructors as public.
But you must remember that a class is a concept in an object-oriented paradigm and the objects are the real-world entities that have ceratin features and a well-defined behavior. Still, there are many applications in which we don’t deal with real-world objects. However, object-oriented languages like C# make it mandatory to write the code in a class only.
To illustrate further, suppose we want to create a class which provides several mathemetical functions. These functions are mostly static and called with the name of class and dot operator. This class of mathematical operationss doesn’t represent any real-world entity. In such case, we should restrict user to create an object of this class. Therefore, we should make the constructor private in such case.
Consider another example of a class that contains functions for computing factorial of a number.
using System;
namespace PrivateConstructorDemo
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine(FactorialFunctions.Factorial(6));
Console.WriteLine(FactorialFunctions.RecursiveFactorial(9));
}
}
class FactorialFunctions
{
public static int Factorial(int v)
{
int fact = 1;
if (v == 0) return 1;
for (int i = 1; i <= v; i++)
{
fact = fact * i;
}
return fact;
}
public static int RecursiveFactorial(int v)
{
if (v == 0)
return 1;
else
return v * RecursiveFactorial(v - 1);
}
}
}
The class FactorialFunctions contains only two static methods. Now, let us create object of this class:
static void Main(string[] args)
{
FactorialFunctions ob = new FactorialFunctions();
Console.WriteLine(FactorialFunctions.Factorial(6));
Console.WriteLine(FactorialFunctions.RecursiveFactorial(9));
}
The above code will compile without error since the compiler provides a default constructor to a class if the programmer doesn’t define one. However, it doesn’t make sense to instantiate this class, since it doesn’t have any non-static member. Therefore, by making the constructor private, we can prevent creating an instance of this class, as shown below.
using System;
namespace PrivateConstructorDemo
{
class Program
{
static void Main(string[] args)
{
// FactorialFunctions ob = new FactorialFunctions();
Console.WriteLine(FactorialFunctions.Factorial(6));
Console.WriteLine(FactorialFunctions.RecursiveFactorial(9));
}
}
class FactorialFunctions
{
FactorialFunctions() { }
public static int Factorial(int v)
{
int fact = 1;
if (v == 0) return 1;
for (int i = 1; i <= v; i++)
{
fact = fact * i;
}
return fact;
}
public static int RecursiveFactorial(int v)
{
if (v == 0)
return 1;
else
return v * RecursiveFactorial(v - 1);
}
}
Now, let us summarize important points regarding the usage of private constructors.
- We can use private constructor in a class if it has no instance member.
- Use of private constructor prevents a class from being inherited.
- Private constructors are used to create a singleton class.
- If a class contains static factory methods to create instance of that class, then it should have private constructors.
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
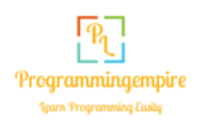