Programmingempire
In this post, I will explain a few Examples of Static Constructors in C#. The static constructors in a class serve the purpose of initializing the static fields in that class. Therefore, if you have the static fields defined in a class, then use the static constructor to initialize them. Since static fields are applicable to all the objects of the class. So it doesn’t make sense to initialize them in a non-static constructor on the creation of an object every time.
Examples of Static Constructors in C#
To illustrate this concept, let us define a class user that represents an online user. Suppose, all the users have a common society email id. As the society email id is common for all users, we declare it as static. However, the login_time is different, each time the user logs in.
If we use the non-static constructor for both static as well as non-static fields, then each time we create an object, the constructor will also initialize the static fields, which we don’t require. Therefore, in the following code, the initialization of the society_email field should happen only once when the object is created.
class user
{
static string society_email;
string login_time;
public user()
{
society_email = "society@gmail.com";
login_time = DateTime.Now.ToString();
}
}
Now, we create a static constructor also and initialize the society_email field in the static constructor as shown below.
class user
{
static string society_email;
string login_time;
static user()
{
society_email = "society@gmail.com";
}
public user()
{
login_time = DateTime.Now.ToString();
}
}
Important Points about Static Constructors in C#
- A static constructor is called only once regardless of how many objects are created.
- We do no call static constructors explicitly like the non-static constructors when we create objects.
- Static constructors are called before any other constructor is called.
- They do not take any parameters.
- The use of any access modifier is not allowed with static constructors.
- We can not overload static constructors.
The following example illustrates these points about static constructors. In this example, we define a class Resident. This class has a static field called societyID, common for all the residents. We initialize this static field in the static constructor. However, a non-static constructor initializes all other non-static fields. In the Main() method, we create several objects of the class Resident. However, the compiler calls the static constructor only once when we create the first object, whereas the compiler calls a non-static constructor every time an object is created, as shown in the output.
namespace StaticConstructorDemo
{
class Program
{
static void Main(string[] args)
{
Resident ob = new Resident();
Console.WriteLine(ob);
Resident ob1 = new Resident();
Console.WriteLine(ob1);
Resident ob2 = new Resident("Vikas", 20);
Console.WriteLine(ob2);
}
}
class Resident
{
static int societyID;
string name;
int age;
static Resident()
{
societyID = 100;
Console.WriteLine("Inside Static Constructor");
}
public Resident()
{
name = "Resident"; age = 1;
Console.WriteLine("Inside Default Constructor");
}
public Resident(string name, int age)
{
this.name = name;
this.age = age;
Console.WriteLine("Inside Parameterized Constructor");
}
public override string ToString()
{
String str = "Society ID: " + societyID + " Resident Name: " + name + " Age: " + age;
return str;
}
}
}
Output:
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
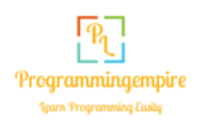