Programmingempire
Object Initializers in C# provide us a way to initialize objects without using constructors.
Examples of Object Initializers in C#
You can use the object initializer syntax to initialize an object as well as to initialize a collection also. In this section, you will find several examples of the usage of object initializers.
Example 1: Initializing an object as well as an array of objects.
The following example shows the use of object initializer syntax in creating the object of MyClass. Evidently, in the absence of a non-static constructor, the instance variables a and b get the initial values by specifying their name explicitly.
Further, in this example, we also create an array of objects and initialize all the elements in the same manner inside the for loop. Finally, we create a generic stack of the type MyClass using the array created earlier. Hence, in this example, we use object initializer syntax to initialize an object, create an array of objects as well as create a generic stack using that array.
using System;
using System.Collections.Generic;
namespace ObjectInitializerDemo
{
class Program
{
static void Main(string[] args)
{
MyClass ob = new MyClass { a = 12, b = 20 };
MyClass[] ob_arr = new MyClass[5];
for (int i = 0; i < 5; i++)
{
ob_arr[i] = new MyClass { a = i + 1, b = i + 5 };
}
foreach (MyClass x in ob_arr)
{
Console.WriteLine(x);
}
Console.WriteLine("nObjects in Stack: ");
Stack<MyClass> st = new Stack<MyClass>(ob_arr);
foreach (MyClass s in st)
{
Console.WriteLine(s);
}
}
}
class MyClass
{
public int a, b;
public override string ToString()
{
String str = "Object Data: " + " a = " + a + " b= " + b;
return str;
}
}
}
Example 2. Creating an object by initializing only a subset of fields at a time.
This example shows how we can initialize an object using a different number of instance variables at a time. In particular, the Emp class contains five instance variables. As shown below, we create several objects of the Emp class in the Main() method. Each object has been initialized with a different number of fields. Moreover, the order of the fields doesn’t matter since the name of the field is also specified when it is assigned a value.
To be sure, if we don’t use object initializer syntax in such situation, then we need to define several parameterized constructors specific to a particular usage.
using System;
namespace ObjectInitializer1
{
class Program
{
static void Main(string[] args)
{
Emp ob1 = new Emp { salary = 10000, ename = "Shridhar" };
Console.WriteLine(ob1);
Emp ob2 = new Emp { empID = 1, ename = "Bansi Lal", department = "Operations", salary = 5000, age = 43 };
Console.WriteLine(ob2);
Emp ob3 = new Emp { ename = "Mohan Lal", department = "Construction" };
Console.WriteLine(ob3);
Emp ob4 = new Emp { empID = 2, ename = "Brij Kishor" };
Console.WriteLine(ob4);
Emp ob5 = new Emp { department = "Technical", empID = 3 };
Console.WriteLine(ob5);
}
}
class Emp
{
public int empID, salary, age;
public String ename, department;
public override string ToString()
{
String str = "Emp ID: "+empID+" Name: "+ename+" Age: "+age+" Department: "+department+" Salary: "+salary;
return str;
}
}
}
Output:

Example 3. Using Object Initializers with Collections
In this example, we use object initializer syntax to initialize several different types of collections available in C# language. First, we create an ArrayList with different types of initial values including the object of class A. In the same manner, we initialize the object of class A itself inside the ArrayList.
Next, we create a Hashtable object and initialize it with different key-value pairs in the same manner.
using System;
using System.Collections;
namespace ObjectInitializer2
{
class Program
{
static void Main(string[] args)
{
ArrayList list1 = new ArrayList { 12, 15, "ABC", 67.9, null, new A { i=1, k= 2,j= 3 }, 89, true, 3.14 };
Console.WriteLine("Elements of ArrayList: ");
foreach (object ob in list1)
Console.Write(ob + " ");
Console.WriteLine();
Hashtable hsh = new Hashtable {
{ "A", 10 },
{ "B", 9.8 },
{ "C", 200 },
{ "D", 385.99 },
{ "E", 27.6 }
};
Console.WriteLine("Hashtable Elements: ");
Console.WriteLine("KEY VALUE");
foreach (DictionaryEntry p in hsh)
Console.WriteLine(p.Key + " " + p.Value);
Console.WriteLine();
}
class A
{
public int i, j, k;
public override string ToString()
{
String str = "Object data: " + i + " " + j + " " + k;
return str;
}
}
}
}
Benefits of Object Initializers in C#
- Object initializer allows us to create objects without using a constructor.
- It is easy to add elements in collections such as Stack, Queue, List, Arrays, etc. using object initializer syntax.
- The code becomes more readable when we use object initializer since we specify the name of the field while creating objects.
- Object Initializer gives us flexibility in specifying the field values in any order.
- We need not specify many overloaded constructors.
- Object initializers are useful in multi-threading.
Drawbacks of Object Initializers
It is sometimes harder to debug the code if an exception arises.
Summary
In this article, several examples of object initializer syntax are provided. Basically, we use this method of initializing the objects when we want to do it quickly and when we need to define several constructors otherwise. This object initializer syntax is applicable for initializing objects, array of objects and collections as well.
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
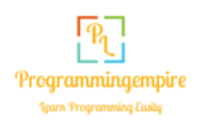