Programmingempire
This article describes Anonymous Functions in C# with examples. Basically, anonymous functions are those functions that don’t have any name. Hence, we define the anonymous functions at that place in the code where we need to use them.
In fact, C# has a delegate keyword that we can use to create anonymous functions. In order to create an anonymous function, we must create a variable of the type delegate. Further, we need to create a function using the delegate keyword. After that, the function is assigned to the variable of the delegate type.
How to Create Anonymous Functions in C#
In order to create an anonymous function first, we need to create a delegate. The following syntax shows how to create a delegate.
delegate return-type delegate-name(parameter-list);
Examples:
delegate int mydelegate(int a, int b);
delegate String mydelegate1(String s1);
After that, we need to create a variable of the delegate type declared earlier. For example,
mydelegate v1, v2;
mydelegate1 x1, x2;
Now that we have variables of these delegate types, we can create anonymous methods of matching signatures and assign them to these variables. For example,
v1=delegate(int a, int b){
//statements
}
Example Program to Demonstrate Anonymous Functions in C#
The following code shows how to create and use anonymous functions in C#. As can be seen, we have two delegate types. The first one returns a value of int type and takes no parameter. Likewise, the second delegate takes an integer type parameter and returns an integer type value.
Calling an Anonymous Method
In order to call an anonymous method, we just need to use the delegate variable name followed by the parentheses. Furthermore, any parameter should be enclosed in the parentheses separated by a comma. Also, assign the returned value in a variable of the appropriate type. For instance, consider the following method call
<return-type> <variable-name> = <delegate-variable-name>(<parameter-list>);
For example,
int x = mydelegate(5, 12);
The following code shows two anonymous functions that return the sum of numbers.
using System;
namespace AnonymousFunctionExample2
{
delegate int d1();
delegate int d2(int n);
class Program
{
static void Main(string[] args)
{
d1 ob = delegate ()
{
int n = 5;
int s = 0;
for(int i=1;i<=n;i++)
{
s += i;
}
return s;
};
int s1 = ob();
Console.WriteLine("Sum = " + s1);
d2 ob1 = delegate (int j)
{
int s = 0;
for (int i = 1; i <= j; i++)
{
s += i;
}
return s;
};
int s2 = ob1(7);
Console.WriteLine("Sum = " + s2);
}
}
}
Output

Further Reading
How to Create Instance Variables and Class Variables in Python
Comparing Rows of Two Tables with ADO.NET
Example of Label and Textbox Control in ASP.NET
One Dimensional and Two Dimensuonal Indexers in C#
Private and Static Constructors in C#
Programs to Find Armstrong Numbers in C#
One Dimensional and Two Dimensional Indexers in C#
Generic IList Interface and its Implementation in C#
Creating Navigation Window Application Using WPF in C#
Find Intersection Using Arrays
An array of Objects and Object Initializer
Performing Set Operations in LINQ
Data Binding Using BulletedList Control
Understanding the Quantifiers in LINQ
Deferred Query Execution and Immediate Query Execution in LINQ
Examples of Query Operations using LINQ in C#
An array of Objects and Object Initializer
Language-Integrated Query (LINQ) in C#
Examples of Connected and Disconnected Approach in ADO.NET
IEnumerable and IEnumerator Interfaces
KeyValuePair and its Applications
Learning All Class Members in C#
Examples of Extension Methods in C#
How to Setup a Connection with SQL Server Database in Visual Studio
Understanding the Concept of Nested Classes in C#
A Beginner’s Tutorial on WPF in C#
Explaining C# Records with Examples
Everything about Tuples in C# and When to Use?
Linear Search and Binary search in C#
Examples of Static Constructors in C#
When should We Use Private Constructors?
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
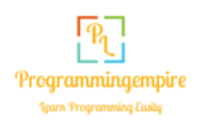