Programmingempire
In general, Data Binding is a way to communicate the data among the code and UI components. Today I will explain How Data Binding Works in WPF (Windows Presentation Foundation). In order to get familiar with WPF, you can go through this basic article on WPF. While creating a navigation window application in WPF is explained here.
To begin with, let us first discuss the concept of data binding. Basically, data binding provides a mechanism that keeps the business logic and UI elements separate while allowing the application to interact with data. In fact, UI elements can fetch data from a variety of sources. Whenever, the application uses data binding, any change in the data source is reflected instantly in UI elements.
Data binding has four elements. The first one is the target object. For instance, if a TextBox displays the data, then TextBox is the target object. The second one is the target property. For example, Text is the target property in the case of a TextBox. The third one is the source object. For instance, the code contains a class called Student, then the object of this class is called the source object. Finally, the fourth one is the source value. In this example, the source value is the Name in the Student object.
The Types of Data Binding in WPF
The following four types of Data Binding are possible in a WPF application.
- One Way Binding
- Two Way Binding
- One Time Binding
- One Way to Source Binding
One Way Binding
Whenever any change occurs in the source property, the same is instantly applies to the target property value. However, the reverse is not true. In other words, changing the value of the target property value doesn’t affect the source property.
Two Way Binding
In contrast to the One Way Binding, in Two Way Binding, the changes are bidirectional. Hence any change in either the source property or in the target property will cause an instant change in the other one.
One Time Binding
In like manner to the One Way Binding, the One Time Binding also propagates the changes from the source property to the target property value. However, it does so only once at the time of initialization. Further changes in the source property value don’t change the target property value.
One Way to Source Binding
Analogous to the One Way Binding, the One Way to Source Binding also causes the updates to take place in one direction. However, now it is from the target to the source. Therefore, whenever the target property value changes, the same is reflected in the source. While the same doesn’t happen in the reverse direction.
Examples of Data Binding
The following examples demonstrate How Data Binding Works in WPF. In general, all the examples will use the following class.
public partial class MainWindow : Window
{
Numbers ob = new Numbers {X=35, Y=97 };
public MainWindow()
{
InitializeComponent();
this.DataContext = ob;
}
private void findsum(object sender, RoutedEventArgs e)
{
String msg = $"Sum of {ob.X} and {ob.Y} is {ob.Sum}";
MessageBox.Show(msg);
}
}
class Numbers
{
int x, y, sum;
public int X
{
get
{
return x;
}
set
{
x = value;
}
}
public int Y
{
get
{
return y;
}
set
{
y = value;
}
}
public int Sum
{
get
{
return x + y;
}
}
}
Example of One Way Binding
<Window x:Class="WpfApp4.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WpfApp4"
mc:Ignorable="d"
Title="Data Binding Example" Height="350" Width="450">
<Grid Margin="10,10,10,10">
<Grid.ColumnDefinitions>
<ColumnDefinition/>
<ColumnDefinition/>
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
</Grid.RowDefinitions>
<Label Margin="2,2,2,2" Grid.Row="0" Grid.Column="0"
Grid.ColumnSpan="2" Background="Beige"
Foreground="DarkBlue" FontSize="20"
HorizontalAlignment="Center">Sum of Two Numbers</Label>
<Label Margin="2,2,2,2" Grid.Row="1" Grid.Column="0"
Background="Beige"
Foreground="DarkBlue" FontSize="20"
HorizontalAlignment="Left">First Number: </Label>
<Label Margin="2,2,2,2" Grid.Row="2" Grid.Column="0"
Background="Beige"
Foreground="DarkBlue" FontSize="20"
HorizontalAlignment="Left">Second Number:</Label>
<TextBox Margin="2,2,2,2" Grid.Row="1" Grid.Column="1"
Background="Beige" Foreground="DarkBlue" FontSize="20"
HorizontalAlignment="Left" Width="200" Name="t1"
Text="{Binding X, Mode=OneWay}"
/>
<TextBox Margin="2,2,2,2" Grid.Row="2" Grid.Column="1"
Background="Beige" Foreground="DarkBlue" FontSize="20"
HorizontalAlignment="Left" Width="200"
Name="t2" Text="{Binding Y, Mode=OneWay}"/>
<Button Margin="2,2,2,2" Grid.Row="3" Grid.Column="0"
Background="Beige" Foreground="DarkBlue" FontSize="20"
Grid.ColumnSpan="2"
HorizontalAlignment="Center" Width="200" Height="40"
Content="Find Sum" Click="findsum"/>
</Grid>
</Window>
Output

Example of Two Way Binding
The following example shows a Two Way Binding in which changing the values of the properties X and Y occur when we change the data in the corresponding TextBox. Also, the values from the properties also get transferred to the corresponding TextBox.
<Window x:Class="WpfApp4.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WpfApp4"
mc:Ignorable="d"
Title="Data Binding Example" Height="350" Width="450">
<Grid Margin="10,10,10,10">
<Grid.ColumnDefinitions>
<ColumnDefinition/>
<ColumnDefinition/>
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
</Grid.RowDefinitions>
<Label Margin="2,2,2,2" Grid.Row="0" Grid.Column="0"
Grid.ColumnSpan="2" Background="Beige"
Foreground="DarkBlue" FontSize="20"
HorizontalAlignment="Center">Sum of Two Numbers</Label>
<Label Margin="2,2,2,2" Grid.Row="1" Grid.Column="0"
Background="Beige"
Foreground="DarkBlue" FontSize="20"
HorizontalAlignment="Left">First Number: </Label>
<Label Margin="2,2,2,2" Grid.Row="2" Grid.Column="0"
Background="Beige"
Foreground="DarkBlue" FontSize="20"
HorizontalAlignment="Left">Second Number:</Label>
<TextBox Margin="2,2,2,2" Grid.Row="1" Grid.Column="1"
Background="Beige" Foreground="DarkBlue" FontSize="20"
HorizontalAlignment="Left" Width="200" Name="t1"
Text="{Binding X, Mode=TwoWay}"
/>
<TextBox Margin="2,2,2,2" Grid.Row="2" Grid.Column="1"
Background="Beige" Foreground="DarkBlue" FontSize="20"
HorizontalAlignment="Left" Width="200"
Name="t2" Text="{Binding Y, Mode=TwoWay}"/>
<Button Margin="2,2,2,2" Grid.Row="3" Grid.Column="0"
Background="Beige" Foreground="DarkBlue" FontSize="20"
Grid.ColumnSpan="2"
HorizontalAlignment="Center" Width="200" Height="40"
Content="Find Sum" Click="findsum"/>
</Grid>
</Window>
Output

Further Reading
How to Create Instance Variables and Class Variables in Python
Comparing Rows of Two Tables with ADO.NET
Example of Label and Textbox Control in ASP.NET
One Dimensional and Two Dimensuonal Indexers in C#
Private and Static Constructors in C#
Programs to Find Armstrong Numbers in C#
One Dimensional and Two Dimensional Indexers in C#
Generic IList Interface and its Implementation in C#
Creating Navigation Window Application Using WPF in C#
Find Intersection Using Arrays
An array of Objects and Object Initializer
Performing Set Operations in LINQ
Data Binding Using BulletedList Control
Understanding the Quantifiers in LINQ
Deferred Query Execution and Immediate Query Execution in LINQ
Examples of Query Operations using LINQ in C#
An array of Objects and Object Initializer
Language-Integrated Query (LINQ) in C#
Examples of Connected and Disconnected Approach in ADO.NET
IEnumerable and IEnumerator Interfaces
KeyValuePair and its Applications
Learning All Class Members in C#
Examples of Extension Methods in C#
How to Setup a Connection with SQL Server Database in Visual Studio
Understanding the Concept of Nested Classes in C#
A Beginner’s Tutorial on WPF in C#
Explaining C# Records with Examples
Everything about Tuples in C# and When to Use?
Linear Search and Binary search in C#
Examples of Static Constructors in C#
When should We Use Private Constructors?
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
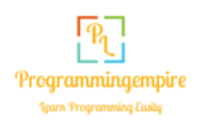