In this blog, I will discuss How to Master Full Stack Development.
Mastering Full Stack Development involves becoming proficient in both front-end and back-end technologies, as well as understanding how they interact with each other to create a seamless user experience. The following practice problems help you learn full-stack development better.
Practice Problems on Full Stack Development
Programs on ES6
- Write a program using arrow function to find square root of elements of an array.
- How to convert string concatenation to template literals?
- Write a program to extract values from an object using destructuring assignment.
- Develop a program to merge arrays using the spread syntax.
Programs on React
- Write a program to display “Hello, World!” using a functional component in React.
- Create a counter app using functional components in React.
- How to render a list of items using the map function in React?
- Develop a program to toggle the visibility of content using state in React.
- Implement a program to change the background color of a component on button click using state in React.
- Create a React component that takes a name prop and displays a greeting message.
- Develop a React component to display a product card, accepting props like productName, price, and imageUrl.
- Build a React component for a todo item, accepting props like task, completed, and onToggle.
- Design a React component to display a list of user profiles, accepting a prop named profiles.
- Develop a React component to create a simple form, accepting props like onSubmit and fields.
- Write a program to fetch and display data from an API using the useEffect hook in React.
- Create a program to manage a simple form with input fields and a submit button using state in React.
- Develop a program to create a basic calculator (addition, subtraction, multiplication, division) using state in React.
- Write a program to create a simple todo list with the ability to add and remove items using state in React.
- Develop a program to create a basic login form with validation using state in React.
Programs on Node.js
- Create a Node.js application to perform various file operations.
- How to append in a text file in Node.js?
- Develop a Node.js application to upload files and save them to a specified directory.
- Write a program to download files using node.js
- Implement a simple HTTP server in Node.js using the built-in http module. The server should listen for incoming requests and respond with basic text or HTML.
Programs on Angular
- Create a Hello World app using angular.
- Implement an app using angular that displays a web page using components
- Create a router app using angular.
- Write a program to demonstrate Angular pipes.
Programs on React Router and React Redux
- Create a router app using react.
- Develop a counter app using react redux.
- Connect to MongoDB database from a Node.js application and print “Connected successfully” to the console.
Programs on MongoDB and Node.js Connectivity
- Insert a document into a MongoDB collection using Node.js.
- Retrieve and print all documents from a MongoDB collection using Node.js.
- Update a document in a MongoDB collection using Node.js.
- Delete a document from a MongoDB collection using Node.js.
- Implement basic CRUD operations (Create, Read, Update, Delete) on a MongoDB collection using Node.js.
- Create a RESTful API using Node.js and Express.js to perform CRUD operations on a MongoDB collection.
- Develop a Node.js program using Express.js to build a RESTful API server. Define routes to handle CRUD operations on resources such as users or products.
Programs on RESTful APIs Using Node.js, Extress.js, and MongoDB
- Implement a RESTful API using Node.js, Express, and MongoDB to handle user authentication. Implement endpoints for user registration, login, logout, and password reset.
- Create a REST API to manage a todo list application. Implement endpoints to create, read, update, and delete tasks. Ensure authentication is required for modifying tasks.
- Develop a RESTful API for managing products in an e-commerce application. Implement endpoints to create, read, update, and delete products. Include features like search and filtering.
Remember, mastering full-stack development is a continuous journey, so stay curious, keep practicing, and never stop learning.
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
100+ MCQs On Java Architecture
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
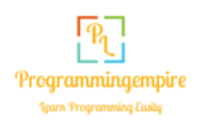