This blog describes how to extract values from an object using destructuring assignment in ES6.
In ES6, you can use destructuring assignment to extract values from an object. For example.
// Object with key-value pairs
const person = {
name: 'Alice',
age: 30,
city: 'Wonderland'
};
// Destructuring assignment
const { name, age, city } = person;
console.log(name); // Output: Alice
console.log(age); // Output: 30
console.log(city); // Output: Wonderland
Output
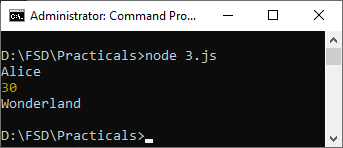
In this example, { name, age, city }
is the destructuring pattern. It extracts the values of name
, age
, and city
properties from the person
object into separate variables with the same names.
You can also provide default values in case the property is undefined
const { name, age, city, country = 'Unknown' } = person;
console.log(country); // Output: Unknown
If you want to rename the variables while destructuring, you can do so.
const { name: personName, age: personAge, city: personCity } = person;
console.log(personName); // Output: Alice
console.log(personAge); // Output: 30
console.log(personCity); // Output: Wonderland
Here, name
, age
, and city
properties are being renamed to personName
, personAge
, and personCity
, respectively, during destructuring.
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
100+ MCQs On Java Architecture
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
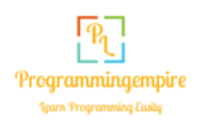