This blog describes How to Find Square Root of Elements of an Array Using Arrow Function in ES6.
You can find the square root of elements in an array using arrow functions in ES6 by utilizing the map()
function along with the arrow function. For example.
// Array of numbers
const numbers = [4, 9, 16, 25];
// Using map() and arrow function to find square roots
const squareRoots = numbers.map(number => Math.sqrt(number));
console.log(squareRoots); // Output: [2, 3, 4, 5]
Output

In this example:
map()
is used to iterate over each element of the array.- The arrow function
(number => Math.sqrt(number))
calculates the square root of each element. Math.sqrt()
is the method used to find the square root of a number.
This will produce a new array squareRoots
containing the square roots of each element in the original array numbers
.
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
100+ MCQs On Java Architecture
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
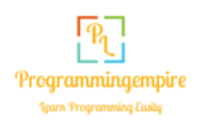