In this blog, I will explain How to Create an Admission Form in Tkinter.
Create a GUI based form for admission purpose for your college.
Creating a GUI-based admission form for a college using Tkinter involves designing a form with various input fields where prospective students can enter their personal information. Here’s an example of how you can create a basic admission form using Tkinter.
import tkinter as tk
from tkinter import ttk
def submit_form():
# Retrieve input values from the entry fields
name = name_entry.get()
age = age_entry.get()
gender = gender_var.get()
course = course_var.get()
address = address_text.get("1.0", "end-1c")
# Display the submitted information
submission_text = f"Name: {name}\nAge: {age}\nGender: {gender}\nCourse: {course}\nAddress: {address}"
submission_label.config(text=submission_text)
# Create the main window
root = tk.Tk()
root.title("College Admission Form")
# Create and pack labels and entry fields for name, age, and gender
tk.Label(root, text="Name:").grid(row=0, column=0, sticky="w")
name_entry = tk.Entry(root)
name_entry.grid(row=0, column=1)
tk.Label(root, text="Age:").grid(row=1, column=0, sticky="w")
age_entry = tk.Entry(root)
age_entry.grid(row=1, column=1)
tk.Label(root, text="Gender:").grid(row=2, column=0, sticky="w")
gender_var = tk.StringVar()
gender_combobox = ttk.Combobox(root, textvariable=gender_var, values=["Male", "Female", "Other"])
gender_combobox.grid(row=2, column=1)
gender_combobox.current(0)
# Create and pack a label and dropdown menu for selecting course
tk.Label(root, text="Course:").grid(row=3, column=0, sticky="w")
course_var = tk.StringVar()
course_combobox = ttk.Combobox(root, textvariable=course_var, values=["Computer Science", "Engineering", "Business", "Medicine"])
course_combobox.grid(row=3, column=1)
course_combobox.current(0)
# Create and pack a label and text area for entering address
tk.Label(root, text="Address:").grid(row=4, column=0, sticky="w")
address_text = tk.Text(root, height=4, width=30)
address_text.grid(row=4, column=1)
# Create a button to submit the form
submit_button = tk.Button(root, text="Submit", command=submit_form)
submit_button.grid(row=5, column=0, columnspan=2)
# Create a label to display the submitted information
submission_label = tk.Label(root, text="")
submission_label.grid(row=6, column=0, columnspan=2)
# Start the Tkinter event loop
root.mainloop()
Output

This code creates a simple admission form with fields for entering the student’s name, age, gender, course choice, and address. When the user clicks the “Submit” button, the entered information is retrieved from the entry fields and displayed below the form.
Further Reading
How to Perform Dataset Preprocessing in Python?
How to Use Generators in Python?
How to Create a Window in Tkinter?
Features and Benefits of Tkinter
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
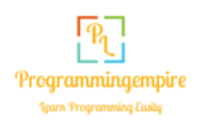