Programmingempire
The following Python Practice Exercise comprises basic programming constructs, control flow, and functions in python. Also, the learner can apply the concepts of data structures like lists, tuples, sets, and dictionaries in python applications. Apart from this, it includes the use of object-oriented programming features of python to develop applications. Additionally, the following Python Practice Exercise aims to make you learn how to use exception handling in applications for error handling and gain the knowledge of NumPy and pandas using Python.
Python Practice Exercise
- To begin with, demonstrate running instructions in an Interactive interpreter and a Python Script.
- Also, write a program to purposefully raise Indentation Error and correct it.
- At first, write a program to print the ‘Hello’ message.
- Also, write a program add.py that takes 2 numbers as input and prints its sum.
- After that, write a program to demonstrate the simple mathematical expression and print the result.
- Also, demonstrate the use of different operators in python.
- In order to demonstrate the use of operators in python, write a program to perform the basic arithmetic operations in python.
- Furthermore, Find the sum of all the primes between two ranges.
- For the purpose of understanding strings in python, write a program to perform the string operation.
Data Structures in Python
- Apart from that write a Python program to demonstrate the use of List, Tuple, Set, and Dictionary.
- Create a program for character counting of a string. Also, store all characters in a dictionary.
- Further, demonstrate the use of split and join functions in the string and trace a birthday using the dictionary.
- Find the mean, median, mode for the given set of numbers in a list using function.
- In order to find duplicates in a list write a function.
- Moreover, demonstrate the use of the inbuilt package in python.
- Create a Python program to demonstrate the use of a user-defined package and import statement.
- For the purpose of understanding exception handling, write an exception handling program in python.
- Demonstrate the use of user-defined exceptions.
Classes and Objects
- To begin with, write a python program to create Class and Object.
- Demonstrate the use of instance variables and methods, and class level attributes.
- Further, extend the functionality of Parent classes using Child class
- Also, demonstrate the use of methods overriding and data hiding using class.
Text Files
- To begin with, write a Python program to read and write a text file.
- Write a Python program to copy the contents of a file to another file.
- Create a Python program that takes a text file as input and returns the number of words of a given text file.
In-Built Packages
- In order to understand the NumPy package, write a program to perform the basic operation on NumPy.
- Write a program to perform the basic operation on pandas.
- Create a program to show the line, scatter and bar chart.
Further Reading
How to Implement Inheritance in Python
Find Prime Numbers in Given Range in Python
Running Instructions in an Interactive Interpreter in Python
Deep Learning Practice Exercise
Deep Learning Methods for Object Detection
Image Contrast Enhancement using Histogram Equalization
Transfer Learning and its Applications
Examples of OpenCV Library in Python
Understanding Blockchain Concepts
Example of Multi-layer Perceptron Classifier in Python
Measuring Performance of Classification using Confusion Matrix
Artificial Neural Network (ANN) Model using Scikit-Learn
Popular Machine Learning Algorithms for Prediction
Long Short Term Memory – An Artificial Recurrent Neural Network Architecture
Python Project Ideas for Undergraduate Students
Creating Basic Charts using Plotly
Visualizing Regression Models with lmplot() and residplot() in Seaborn
Data Visualization with Pandas
A Brief Introduction of Pandas Library in Python
A Brief Tutorial on NumPy in Python
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
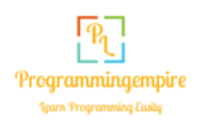