The following article demonstrates How to Use Decorators in Python.
Basically, decorators in Python are a powerful and flexible way to modify or enhance the behavior of functions or methods without changing their actual code. They are often used for tasks like logging, authentication, caching, and more. In fact, decorators are themselves functions that wrap other functions or methods. The following examples demonstrate how you can use decorators in Python.
Creating a Simple Decorator
In order to create a decorator, you define a function that takes another function as its argument and returns a new function that usually extends or modifies the behavior of the original function. The following code shows the syntax for a decorator.
def my_decorator(func):
def wrapper():
# Code to run before the original function
print("Something is happening before the function is called.")
func() # Call the original function
# Code to run after the original function
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
In this example, my_decorator
is a simple decorator that adds some functionality before and after calling the say_hello
function. When you use the @my_decorator
syntax above say_hello
, it’s equivalent to calling say_hello = my_decorator(say_hello)
.
Decorating Functions with Arguments
Additionally, if you want to decorate functions that take arguments, you can make your decorator more flexible using *args
and **kwargs
.
def argument_decorator(func):
def wrapper(*args, **kwargs):
print("Something is happening before the function is called.")
result = func(*args, **kwargs) # Call the original function with its arguments
print("Something is happening after the function is called.")
return result
return wrapper
@argument_decorator
def greet(name):
print(f"Hello, {name}!")
greet("Alice")
Chaining Multiple Decorators
Furthermore, you can apply multiple decorators to a single function by stacking them using the @
symbol. Also, decorators are applied from the innermost to the outermost.
def decorator1(func):
def wrapper():
print("Decorator 1 before")
func()
print("Decorator 1 after")
return wrapper
def decorator2(func):
def wrapper():
print("Decorator 2 before")
func()
print("Decorator 2 after")
return wrapper
@decorator1
@decorator2
def my_function():
print("My function")
my_function()
In this case, my_function
is first wrapped by decorator2
, and then the resulting function is wrapped by decorator1
.
Common Use Cases
In general, decorators are used for various purposes in Python applications. For instance, we can use them in following situations.
- Logging. Adding logging statements before and after function calls.
- Authentication. Similarly, you can use them to check if a user is authorized to access a particular function or resource.
- Caching. Also, we can use them for storing and returning the results of expensive function calls to improve performance.
- Timing. Measuring the execution time of functions.
- Validation. Similarly, for checking input arguments or return values for validity.
In conclusion, decorators are a powerful tool in Python for code reusability and maintainability. Because they allow you to separate concerns and apply cross-cutting behavior to functions and methods without modifying their core logic.
Further Reading
How to Perform Dataset Preprocessing in Python?
Spring Framework Practice Problems and Their Solutions
How to Implement Linear Regression from Scratch?
How to Use Generators in Python?
Getting Started with Data Analysis in Python
Wake Up to Better Performance with Hibernate
Data Science in Insurance: Better Decisions, Better Outcomes
Breaking the Mold: Innovative Ways for College Students to Improve Software Development Skills
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
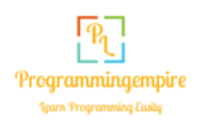