The following code example demonstrates how to Program to Create a List of Numbers Which is Either Divisible By 2 or 4 in Python.
Here’s a program to create a list of numbers from 1 to 20 that are either divisible by 2 or divisible by 4, without using the filter function in Python.
# Create an empty list to store the numbers result = [] # Iterate over the numbers from 1 to 20 for i in range(1, 21): # Check if the number is divisible by 2 or 4 if i % 2 == 0 or i % 4 == 0: # If the condition is true, add the number to the list result.append(i) # Print the final list print(result)
In this program, we use a for loop to iterate over the numbers from 1 to 20. For each number, we check if it’s divisible by 2 or 4 using the modulo operator (%
). If the number is divisible by 2 or 4, we add it to the list result
. Finally, we print the list result
to get the list of numbers from 1 to 20 that are either divisible by 2 or divisible by 4.
Further Reading
Examples of OpenCV Library in Python
A Brief Introduction of Pandas Library in Python
A Brief Tutorial on NumPy in Python
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
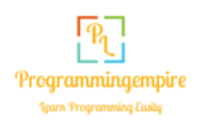