The following programs demonstrate some Examples of Using Loops in Python.
Like other programming languages, python also has a for loop. The following loop varies the variable x from 0 to 34 and prints x multiplied by 3.
for x in range(35):
print(3*x, end=", ")
Output
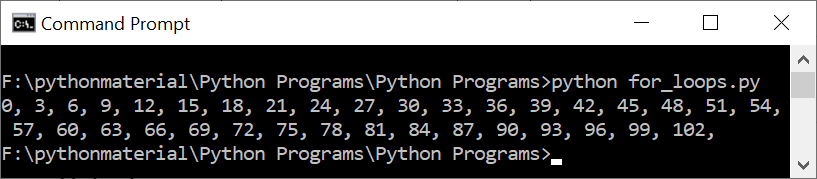
Another example of using for loop is here. The following code prints each element of the list four times.
a = ['Sam', 'Joe', 'Luke']
for user_name in a:
print(user_name*4)
Output
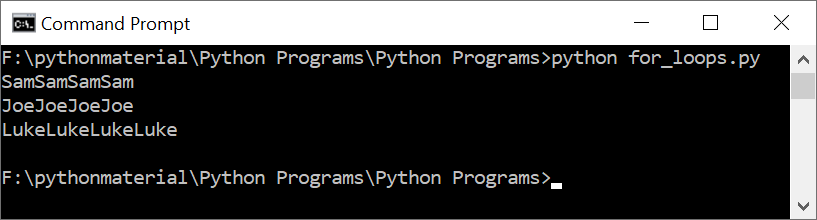
Similarly, we can use a for loop to print a series. The following code displays a series from 12 to 0 with each term of the series decremented by three.
my_nn = 12
while my_nn >= 0:
print(my_nn)
my_nn -= 3
Output
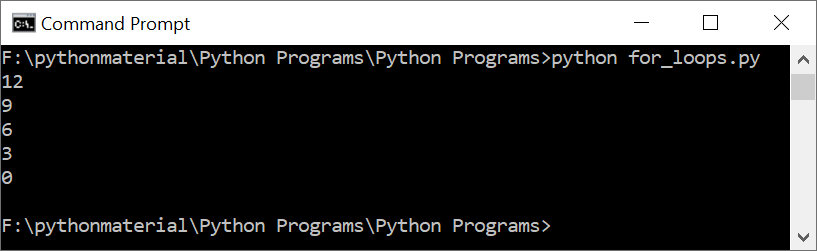
The following loop demonstrates the use of break statement. When i becomes greater than 14, the loop terminates.
for i in range(20):
if i > 14:
break
print(i)
Output

Likewise, the following code demonstrates the use of continue statement. When i becomes equal to 7, the corresponding iteration skips the rest of the statements. Afterward, the loop continues. Hence, the loop all prints all numbers from 0 to 13, except the number 7.
for i in range(14):
if i == 7:
continue
print(i)
Output

Further Reading
Examples of OpenCV Library in Python
A Brief Introduction of Pandas Library in Python
A Brief Tutorial on NumPy in Python
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
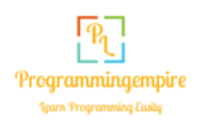