The following program creates a list of numbers in the range 1 to 10 and Remove All Even Numbers from a List in Python. After that print the final list.
# Create a list of numbers from 1 to 10
numbers = list(range(1, 11))
print("Original list: ", numbers)
# Create a new list to store the odd numbers
odd_numbers = []
# Iterate over the numbers in the list
for number in numbers:
# Check if the number is odd
if number % 2 != 0:
# If the number is odd, add it to the new list
odd_numbers.append(number)
# Replace the original list with the new list of odd numbers
numbers = odd_numbers
# Print the final list
print("Final list: ", numbers)
In this program, we first create a list of numbers from 1 to 10 using the range
function and a list
typecast. Then, we create a new list odd_numbers
to store the odd numbers. We use a for loop to iterate over the numbers in the original list and check if each number is odd using the modulo operator (%
). If the number is odd, we add it to the new list odd_numbers
. Finally, we replace the original list with the new list odd_numbers
and print the final list.
Further Reading
Examples of OpenCV Library in Python
A Brief Introduction of Pandas Library in Python
A Brief Tutorial on NumPy in Python
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
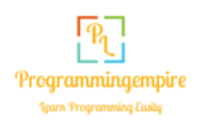