The following code shows an Example of querySelectorAll() Method in JavaScript.
Programmingempire
Illustrating an Example of querySelectorAll() Method in JavaScript
Unlike the querySelector() method, this method selects all elements belonging to a certain class or id.
<html>
<head>
<title>querySelectorAll Method Demo</title>
<script>
function f1()
{
var x1=document.querySelectorAll(".c1");
for(i=0;i<x1.length;i++)
x1[i].style.background="#ffaa22";
}
function f2()
{
var x2=document.querySelectorAll("#d1");
for(i=0;i<x2.length;i++)
x2[i].style.background="#aaff22";
}
</script>
<style>
.c1{
border: 4px solid #33ff99;
border-radius: 10px;
background-color: #5566aa;
color: #aaffff;
font-size: 20px;
text-align: center;
margin:5px;
padding: 10px;
}
.a1{
margin: 50px;
}
.c2{
background-color: #ddeeff;
color: #ff5566;
font-size: 25px;
text-align: left;
margin:40px;
padding: 5px;
width: 800px;
}
</style>
</head>
<body>
<div id="d1" class="c2"><br/><br/>
Example of querySelectorAll() Method<br/>
<br/><br/>
<button id="b1" class="c1">Button 1</button>
<button id="b2" class="c1">Button 2</button>
<button id="b3" class="c1">Button 3</button>
<br/><br/>
<button id="b4" class="c1">Button 4</button>
<button id="b5" class="c1">Button 5</button>
<button id="b6" class="c1">Button 6</button><br/><br/>
<button id="b7" class="c1" onclick="f1()">Change Background of Button</button>
<button id="b8" class="c1" onclick="f2()">Change Background of Div</button>
</div>
</body>
</html>
Output
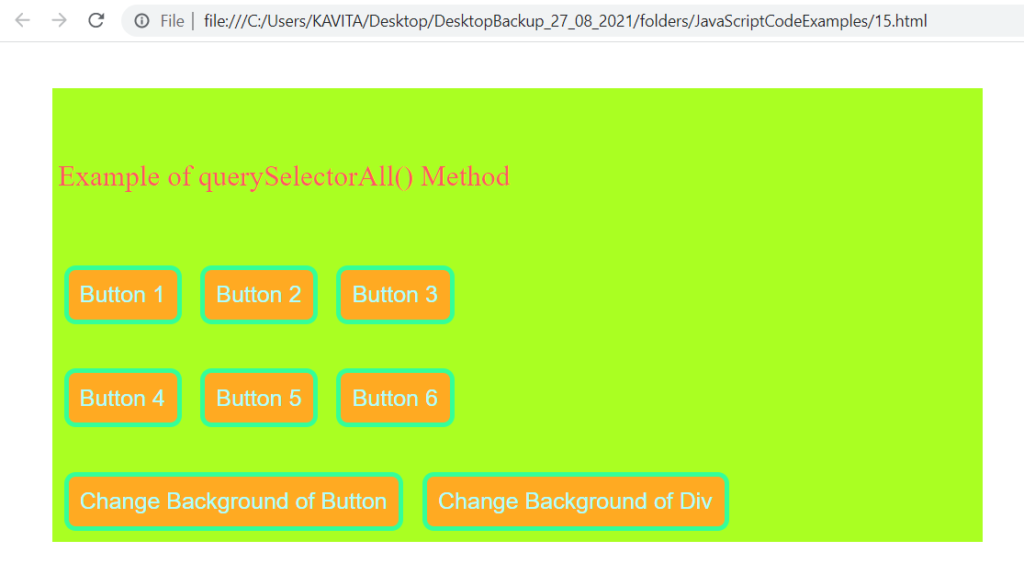
Further Reading
Evolution of JavaScript from ES1 to ES2020
Introduction to HTML DOM Methods in JavaScript
Creating User-Defined Functions in javaScript
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
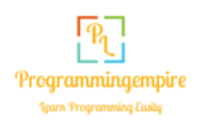