The following code shows an Example of insertAdjacentHTML() Method in JavaScript.
Programmingempire
Illustrating an Example of insertAdjacentHTML() Method in JavaScript
Basically, in the following example, there are four buttons. While the click event handler function of each button inserts an HTML element at a specific position.
<html>
<head>
<title>insertAdjacentHTML Method Demo</title>
<script>
function f1()
{
var v1=document.getElementById("d1");
v1.insertAdjacentHTML('afterend','<div id="d2" class="c1" style="width:350px;">Another Div</div>');
}
function f2()
{
var v1=document.getElementById("d1");
v1.insertAdjacentHTML('afterbegin','<button id="b2" class="c2" style="width:150px;">Another Button</button>');
}
function f3()
{
var v1=document.getElementById("d1");
v1.insertAdjacentHTML('beforebegin','<p id="p2" class="c2">A Dynamically Created Paragraph....</p>');
}
function f4()
{
var v1=document.getElementById("d1");
v1.insertAdjacentHTML('beforeend','<ul id="l1" class="c2" style="width:50px;"><li>one</li><li>two</li><li>three</li></ul>');
}
</script>
<style>
.c1{
border: 4px solid #33ff99;
border-radius: 10px;
background-color: #5566aa;
color: #aaffff;
font-size: 20px;
text-align: center;
margin:5px;
padding: 10px;
}
.a1{
margin: 50px;
}
.c2{
background-color: #ddeeff;
color: #ff5566;
font-size: 25px;
text-align: left;
margin:40px;
padding: 5px;
width: 800px;
}
</style>
</head>
<body>
<div id="d1" class="c2"><br/><br/>
Example of insertAdjacentHTML() Method<br/>
<br/><br/>
<button id="b1" class="c1" onclick="f1()">Add a Div</button>
<button id="b2" class="c1" onclick="f2()">Add a Button</button>
<button id="b3" class="c1" onclick="f3()">Add a Paragraph</button>
<button id="b4" class="c1" onclick="f4()">Add a List</button>
</div>
</body>
</html>
Output
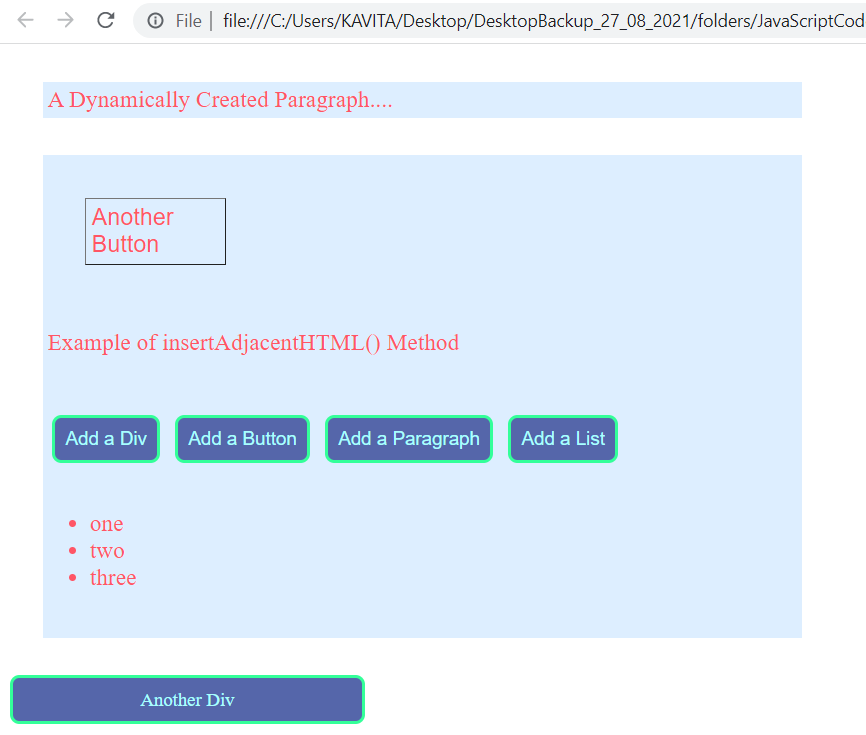
Further Reading
Evolution of JavaScript from ES1 to ES2020
Introduction to HTML DOM Methods in JavaScript
Creating User-Defined Functions in javaScript
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
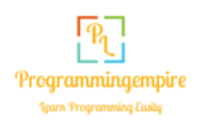